Thermistors are simple, inexpensive, and accurate components that make it easy to get temperature data for your projects. Remote weather stations, home automation systems, and equipment control and protection circuits are some applications where thermistors would be ideal. They’re analog sensors, so the code is relatively simple compared to digital temperature sensors that require special libraries and lots of code.
In this article, I’ll explain how thermistors work, then I’ll show you how to set up a basic thermistor circuit with an Arduino that will output temperature readings to the serial monitor or to an LCD.
Watch the video for this tutorial here:
How a Thermistor Works
Thermistors are variable resistors that change their resistance with temperature. They are classified by the way their resistance responds to temperature changes. In Negative Temperature Coefficient (NTC) thermistors, resistance decreases with an increase in temperature. In Positive Temperature Coefficient (PTC) thermistors, resistance increases with an increase in temperature.
NTC thermistors are the most common, and that’s the type we’ll be using in this tutorial. NTC thermistors are made from a semiconducting material (such as a metal oxide or ceramic) that’s been heated and compressed to form a temperature sensitive conducting material.
The conducting material contains charge carriers that allow current to flow through it. High temperatures cause the semiconducting material to release more charge carriers. In NTC thermistors made from ferric oxide, electrons are the charge carriers. In nickel oxide NTC thermistors, the charge carriers are electron holes.
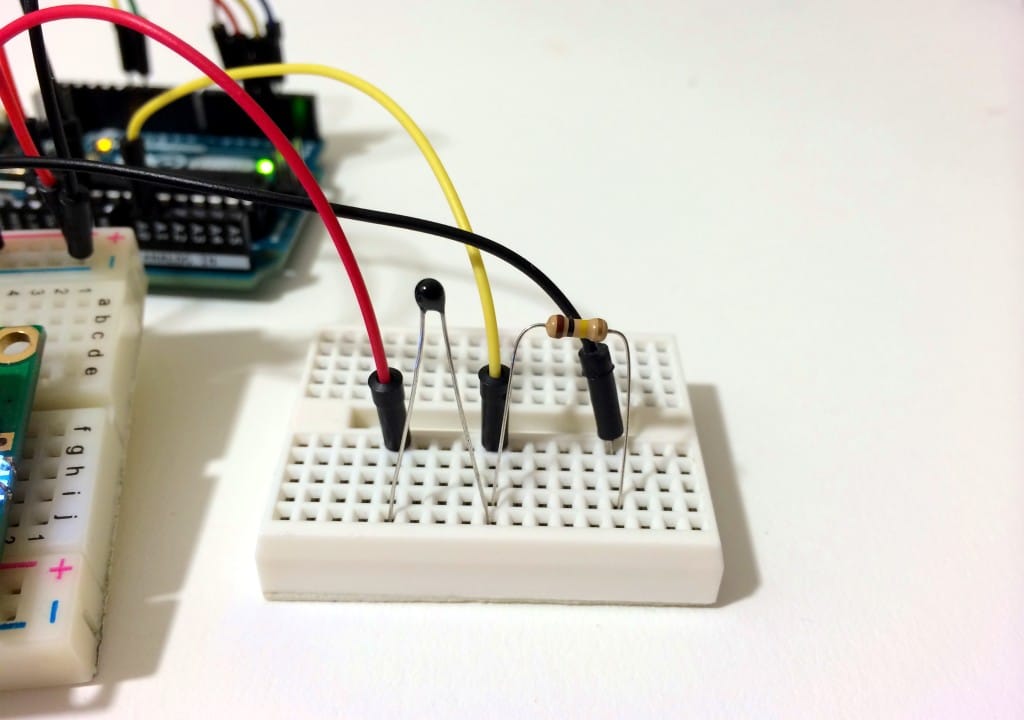
A Basic Thermistor Circuit
Let’s build a basic thermistor circuit to see how it works, so you can apply it to other projects later.
Since the thermistor is a variable resistor, we’ll need to measure the resistance before we can calculate the temperature. However, the Arduino can’t measure resistance directly, it can only measure voltage.
The Arduino will measure the voltage at a point between the thermistor and a known resistor. This is known as a voltage divider. The equation for a voltage divider is:
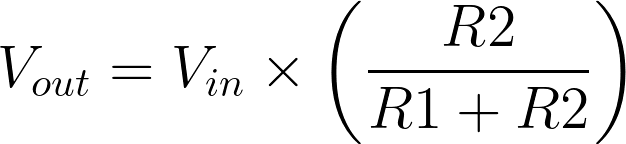
In terms of the voltage divider in a thermistor circuit, the variables in the equation above are:

This equation can be rearranged and simplified to solve for R2, the resistance of the thermistor:
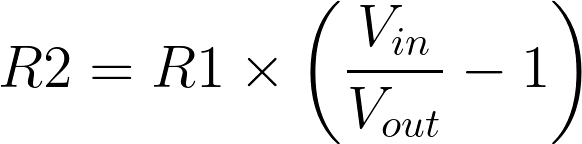
Finally, the Steinhart-Hart equation is used to convert the resistance of the thermistor to a temperature reading.
Connect the Circuit
Connect the thermistor and resistor to your Arduino like this:
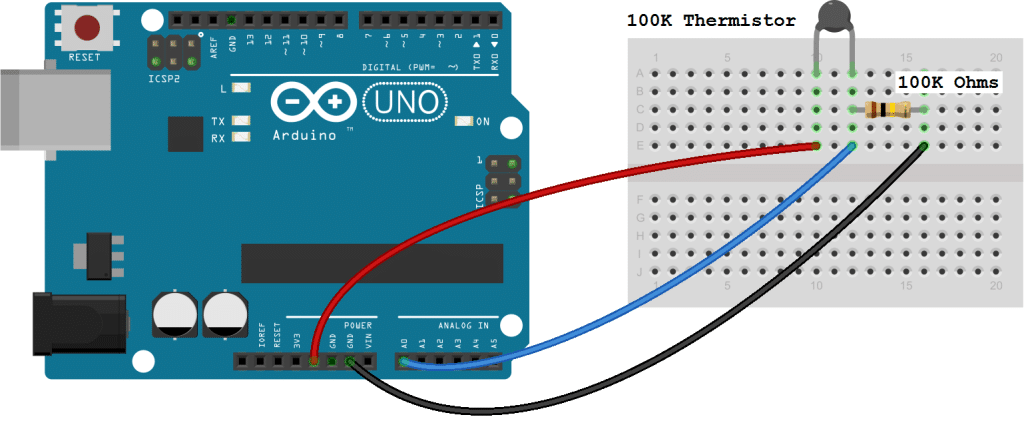
The value of the resistor should be roughly equal to the resistance of your thermistor. In this case, the resistance of my thermistor is 100K Ohms, so my resistor is also 100K Ohms.
The manufacturer of the thermistor might tell you it’s resistance, but if not, you can use a multimeter to find out. If you don’t have a multimeter, you can make an Ohm meter with your Arduino by following our Arduino Ohm Meter tutorial. You only need to know the magnitude of your thermistor. For example, if your thermistor resistance is 34,000 Ohms, it is a 10K thermistor. If it’s 340,000 Ohms, it’s a 100K thermsitor.
Code for Serial Monitor Output of Temperature Readings
After connecting the circuit above, upload this code to your Arduino to output the temperature readings to the serial monitor in Fahrenheit:
int ThermistorPin = 0;
int Vo;
float R1 = 10000;
float logR2, R2, T;
float c1 = 1.009249522e-03, c2 = 2.378405444e-04, c3 = 2.019202697e-07;
void setup() {
Serial.begin(9600);
}
void loop() {
Vo = analogRead(ThermistorPin);
R2 = R1 * (1023.0 / (float)Vo - 1.0);
logR2 = log(R2);
T = (1.0 / (c1 + c2*logR2 + c3*logR2*logR2*logR2));
T = T - 273.15;
T = (T * 9.0)/ 5.0 + 32.0;
Serial.print("Temperature: ");
Serial.print(T);
Serial.println(" F");
delay(500);
}
To display the temperature in degrees Celsius, just comment out line 18 by inserting two forward slashes (“//”) at the beginning of the line.
This program will display Celsius and Fahrenheit at the same time:
int ThermistorPin = 0;
int Vo;
float R1 = 10000;
float logR2, R2, T, Tc, Tf;
float c1 = 1.009249522e-03, c2 = 2.378405444e-04, c3 = 2.019202697e-07;
void setup() {
Serial.begin(9600);
}
void loop() {
Vo = analogRead(ThermistorPin);
R2 = R1 * (1023.0 / (float)Vo - 1.0);
logR2 = log(R2);
T = (1.0 / (c1 + c2*logR2 + c3*logR2*logR2*logR2));
Tc = T - 273.15;
Tf = (Tc * 9.0)/ 5.0 + 32.0;
Serial.print("Temperature: ");
Serial.print(Tf);
Serial.print(" F; ");
Serial.print(Tc);
Serial.println(" C");
delay(500);
}
Code for LCD Output of Temperature Readings
To output the temperature readings to a 16X2 LCD, follow our tutorial, How to Set Up an LCD Display on an Arduino, then upload this code to the board:
#include <LiquidCrystal.h>
int ThermistorPin = 0;
int Vo;
float R1 = 10000;
float logR2, R2, T;
float c1 = 1.009249522e-03, c2 = 2.378405444e-04, c3 = 2.019202697e-07;
LiquidCrystal lcd(12, 11, 5, 4, 3, 2);
void setup() {
Serial.begin(9600);
}
void loop() {
Vo = analogRead(ThermistorPin);
R2 = R1 * (1023.0 / (float)Vo - 1.0);
logR2 = log(R2);
T = (1.0 / (c1 + c2*logR2 + c3*logR2*logR2*logR2));
T = T - 273.15;
T = (T * 9.0)/ 5.0 + 32.0;
lcd.print("Temp = ");
lcd.print(T);
lcd.print(" F");
delay(500);
lcd.clear();
}
Here’s a video of the temperature sensor so you can watch me set it up and see how it works:
Well, that’s about it. Just leave a comment below if you have any questions about this project. And if you like our articles here at Circuit Basics, subscribe and we’ll let you know when we publish new articles. Also, feel free to share this if you know anyone that would find it helpful!
thank you very much
Is connecting wires mean arduino 40 pin female to female jumper connector?
Hi,
I’ve seen your Videos on Youtube and came to your website. Great!
I tried to do make the circuit with the Thermistor (81210W26)and had a strange problem.
I followed the instructions and put the same resistance as the thermistors resistance worth but the Temperature goes down instead to increase when I warm it up. I tried to invert the Thermistor and gained the knowledge that this doesn’t effect it. The temperature of the room is displayed correctly (I have 25°C and the Thermistor displays 25°C)
Also the Temperature goes down to slowly. Exemple: if I have 25°C in the room and take the thermistor in the hand, it decreases only 2°C although I’m still alive. :o)
Have you got an Idea where I have to search for the problem?
Thank you very much for your help.
Phil
Hi Phil,
I couldn’t find any information on your specific thermistor, but it sounds like the problem could be related to if your thermistor is PTC (positive temperature coefficient) or NTC (negative temperature coefficient). The thermistor I used in this article is a NTC thermistor, so if you have a PTC thermistor, it could cause the temperature changes to become opposite from what you would expect. I don’t have a PTC thermsitor to experiment with, so I’m not sure what the code would look like for a PTC thermistor.
Hello to everyone, a little help please? I want to build a thermistor temp sensor using arduino (as in above circuit) but then need to convert measured temperature in degrees centigrade to air flow in meters per second, and be able to display on screen, and record this via computer. Any help suggestions greatly appreciated.
regards
Andrew
only before line Number 8 put double slash (//) like that exmple
// Temp = (Temp * 9.0)/ 5.0 + 32.0;
Jesus man thanks a lot you are savivor :D :D
Perhaps a bit late, but I experienced the same problem. I reversed the + and – leads and this solved it, leaving the center lead the same. Not sure what that had to do with anything, but it worked for me.
I was wondering in the video, how did you get the lcd to work without a potentiometer?
line 14, change R1 * (102… to R1 / (102…
I’m also facing similar problem. When hold the thermistor is temperature getting reduced. Have u find the solution to above issue
i need a heat temperature sensor but it is very expensive. i’m working on a project and i need to heat a metal up to 150 degrees to test it on my machine cooler project. Is this thermistor temperature sensor can measure up to 150 degrees? I am looking forward to your response. Thank you very much.
Hi Krissy, the operating temperature range for this thermistor is -50~+260°C
hotmistor
I tried the project with the LCD. The value read is double than the real value in the room. So on line 10 for LCD I did : ” Temp = (Temp – 273.15)/2; ” and I had the normal value that was supposed to be.
Probably I will have to study this equation.
Or maybe there is something else?
You might try to check the value of the resistor you are using!bc i was using a 220 ohm resistor. And by changing the “R” value you will get the correct readings without changing the calculation part of the program.
Hello, I math.h download? Where can I download it?
hello i have had the same question ,,, but after some research i found out that you dont need to download it it and that it already comes with the arduino IDE …hope this helped
Eventhough im using math.h headefile in my test file im getting the error.
The error is
” /tmp/cc8vrcYJ.o: In function `ThermistorF’:
test1.c:(.text+0x7c): undefined reference to `log’
/tmp/cc8vrcYJ.o: In function `ThermistorC’:
test1.c:(.text+0x284): undefined reference to `log’
collect2: error: ld returned 1 exit status ”
what is the solution for this problem. Im using the same concept for other controller. The code is littile bit different accroding to the controller but it showing error at reading log function from math.h.
What is the solution for this.
Give me reply ASAP.
I rectified that error. Now my problem is as im increasing the temperature the value which is showing on the LCD is decreasing im not getting why this is happening. Here i am using NTC (negative temperature coefficient) thermistor p103. Can any one tell me how to rectify this problem.
please help me
how can i add this alarm
Hi There!
it works brilliantly but could you suggest me how to put 2 thermistors that give 2 readings simultaneously in Celsius?
Hi ….
could you suggest me how to put 3 thermistors that give 3 readings simultaneously in Celsius?.. im trying to connect from 2 weeks but im not able to.please help me.
Hello! I have a question about Steinhart-Hart equasion. In the first code in the fifth line, here: Temp = log(10000.0*((1024.0/RawADC-1))); I don´t understand why we have to use “RawADC-1”? Why we have to do that minus one? Can anyone give me a good answer? Thanks
It has do with the math… check out Adafruit’s page on thermistors
https://learn.adafruit.com/thermistor/using-a-thermistor
First it’s not “RawADC-1” but rather (1024.0/RawADC)-1 since the multiplication takes precedence over the subtraction.
Actually it should really be 1023 instead of 1024 since you can only read from 0 to 1023 from the port.
Or if you prefer it’s (1023.0-RawADC)/RawADC which can directly be deducted from wiring the NTC to the 5V and R1 to the GND.
If you connect it backward (NTC to GND and R1 to 5V) then the equation would be
R2 = R1 * RawADC / (1023.0 – RawADC) however, in this case you might want to use 1024 to avoid dividing by zero if your NTC opens up or get disconnected.
Muhamed Boshra Serag Eldin
didnt understand line 5 and 6.can anyone explain whats going on there?
hi, I am using a 100k glass bead thermistor (meant for 3d printing), and wired it up like you showed and uploaded the code. when i visit the serial monitor the temperature given there is negative and makes no sense whatsoever . Please tell me what is happening.
Same here…..
hi, if you are still interested use 100K thermistor and use the following co-efficience c1 =0.7203283552e-3 c2= 2.171656865e-4 and c3 = 0.8706070062e-7. You can also calculate your own three resistors values and put the on the following link it will calculate the co-efficiece for you http://www.thinksrs.com/downloads
I had the same problem, just for the heck of it, I tried moving the vin from 3.3 volts to 5 volts and suddenly it all began working. where I was getting 456 F I am now getting 74 F Perhaps these glass rod sensor’s are very voltage sensitive.
hi , can this sensor mesure temperature for health ???
sorry about my bad english ! thanks
NTC-MF52AT 10K 3950 equation matching range:
http://www.rixratas.ee/jaga/jaga.php?fn=NTC_MF52AT_10K.jpg
Hello, Yesterday I did it correctly and temperature went up upon touch nevertheless, today The measurement goes down when touched, any idea of what could have happened? I have been using the same resistors and thermistor
what do you call the digital monitor that is small in the video
Thanks a million for the video and sketch. I am building a temp sensor for our local paranormal group (no I am not into ghosts, but my niece is, so this is for her.) So what I need to do, is store the initial temp in a variable, then check the subsequent temps against that temp, and besides reporting the temp, report on the next line weather temps rise or fall, as well as light a red LED if the temp rises, a blue LED if it falls, and a green LED if it remains the same. Should be easy to set this up by editing your sketch, I think, oh and a main switch to turn this unit off and on, as well as hooking up say a 9v battery for power to the unit, again simple, but as I go on, it seems to be more and more complicated.
Arduino: 1.6.11 (Windows 10), Board: “Arduino/Genuino Uno”
Sketch uses 4,176 bytes (12%) of program storage space. Maximum is 32,256 bytes.
Global variables use 222 bytes (10%) of dynamic memory, leaving 1,826 bytes for local variables. Maximum is 2,048 bytes.
avrdude: ser_open(): can’t open device “\\.\COM1”: The system cannot find the file specified.
Problem uploading to board. See http://www.arduino.cc/en/Guide/Troubleshooting#upload for suggestions.
This report would have more information with
“Show verbose output during compilation”
option enabled in File -> Preferences.
I need help.
I want to know which is the maximum operating temperature resistors and capacitors for Arduino uno?
To add more thermistors keep adding the following code and change the analogue read pin. Note that you cannot connect your aditional thermistors to the same voltage supply unless you change the mathematical equation as you will change the resistance of the circuit each time you add a thermistor.
—————————————–
valF=analogRead(1);
tempF=ThermistorF(valF);
valC=analogRead(1);
tempC=ThermistorC(valC);
Serial.print(“Temperature = “);
Serial.print(tempC);
Serial.println(” C”);
delay(1000);
—————————————-
valF=analogRead(2);
tempF=ThermistorF(valF);
valC=analogRead(2);
tempC=ThermistorC(valC);
Serial.print(“Temperature = “);
Serial.print(tempC);
Serial.println(” C”);
delay(1000);
—————————————-
valF=analogRead(3);
tempF=ThermistorF(valF);
valC=analogRead(3);
tempC=ThermistorC(valC);
Serial.print(“Temperature = “);
Serial.print(tempC);
Serial.println(” C”);
delay(1000);
In your video you have used 100k ohm resistor with thermister but in the other part of the video you have used two other resistors with 16×2 lcd !! So my problem is that you have not declare the resistor’s quality and its number in the start of video !! so plz mention the type of these two resistors quickly because it is my project THANKYOU !!
Hi, those resistors set the lcd’s backlight brightness and contrast. They can be a range of values, but potentiometers are probably best to use there. Check out this article for more info: https://www.circuitbasics.com/how-to-set-up-an-lcd-display-on-an-arduino/
Great tutorial. I wonder if it is possible to use a potmeter instead of a thermistor, so I could simulate the temperatures ?
Absolutely, thermistors are basically just variable resistors, like potentiometers. Instead of using the voltage divider, just connect the signal wire to the center pin, and the positive and negative wires to the outside pins…
Please tell me: why this code can not compile with Energia?
Error compiling: undifined reference to ‘log’
collect2: Id returned1 exit status
Thanks so much!
Finally, a good quality video, and no BS waste of time, on Arduino setup and programming. Thanks!
Hello I do not have the data sheet of my thermistor how to dO MY PROGRAM?
hi…anyone please help me…hopefully there is someone who can tell me…
can I know what is the actually thermistor sensor….it is sensor for human body temperature or environment
The thermistor used in this article is mainly used for sensing environmental temperatures. It could be used for human body temperatures, but it depends on how you want to measure the body temperature. Since the human skin acts as a thermal insulator, the temperature of the skin isn’t an accurate representation of actual body temperature. That’s why most body temperatures are taken with an oral thermometer. The problem with using this thermistor orally is that the exposed leads of the thermistor would be partially shorted by the saliva in the mouth. But that said, I have seen some thermistors that have the leads insulated with plastic so those could be used in aqueous environments. Also, you could use this thermistor to take body temperature from the armpit, which is pretty close to the actual body temperature. Hope that helps!
Hi.
I have just made it and is working very well and very fast.
Now i will try to put together with a lcd keypad shield nad a relay and make a thermostat.
But i would like to ask if its possible to the
change the thermometer value with the shield buttons .
So if i want to make any changes i will not need the use of a pc or laptop.
Than you for all tutorials.
Great presentation.
Pol
¿Se puede cambiar ese termistor por un RTD de platino (WZP – PT100) de 100ohms?
Most excellent tutorial. Exactly what I needed to complete my project to read temperature and display on a little OLED display.
What if I want to use more than one thermistor to sense different temperatures, say 10.
How will the schematic and code change? Any ideas for the new circuit?
And if you want to connect more than one sensor, what code you will need to write?
hi
R1=10000 in your example. is not wrong because you are used 100k resistor? if you use 100k resistor R1 must be = 100000?
thank you
I too have the same doubt
hi, if you are still interested use 100K thermistor and use the following co-efficience c1 =0.7203283552e-3 c2= 2.171656865e-4 and c3 = 0.8706070062e-7. You can also calculate your own three resistors values and put the on the following link it will calculate the co-efficiece for you http://www.thinksrs.com/downloads
cheers
unfortunately the link does not work anymore.
via wikipedia you can find an online and offline calculator.
for standart 100K 3d printer thermistors you can use the following settings:
float c1=0.003517835373043556, c2=-0.0002577063055439601, c3=0.000001766946404565146; //c values berekend op https://sanjit.wtf/Calibrator/webCalibrator.html
the included link is to the online calculator.
Steinhart-hart calculator URL updated June 28, 2022: https://www.thinksrs.com/downloads/programs/Therm%20Calc/NTCCalibrator/NTCcalculator.htm
We want to have a continuous record of body temperature instead of room temperature. Which thermistor should we use and how to connect it with arduino?
thanks for this article
how did u write 10k instead of 100k?
I followed the above steps exactly, however the numbers I get are negative 459 Fahrenheit and does not change at all. the only difference in the parts used is the thermistor. I believe its resistance is 24000 ohms and my resistor is 10kohms.
I have noticed you don’t monetize your website, don’t waste your traffic,
you can earn additional cash every month because you’ve got
hi quality content. If you want to know how
to make extra money, search for: Mertiso’s tips best adsense alternative
Can an one help me plzzz? I need to add a Bluetooth transmitter to my temp sensor program to inform me when the temp increases to 60 degrees . an help would be really appreciated. thanks
Good
Any answer to the 10000 vs 100000 R1 resistor value in equation? I’ built it with This part, as you part is out of stock:
https://www.amazon.com/dp/B06XR1TG5N/ref=sspa_dk_detail_2?psc=1
I’d like to find coefficients for it. Would they be the same?
Thanks, nice article!
hi, if you are still interested use 100K thermistor and use the following co-efficience c1 =0.7203283552e-3 c2= 2.171656865e-4 and c3 = 0.8706070062e-7. You can also calculate your own three resistors values and put the on the following link it will calculate the co-efficiece for you http://www.thinksrs.com/downloads
it works for me
The link does not work
Do you have an updated link?
unfortunately the link does not work anymore.
via wikipedia you can find an online and offline calculator.
for standart 100K 3d printer thermistors you can use the following settings:
float c1=0.003517835373043556, c2=-0.0002577063055439601, c3=0.000001766946404565146; //c values berekend op https://sanjit.wtf/Calibrator/webCalibrator.html
the included link is to the online calculator.
Here is the current URL: https://www.thinksrs.com/downloads/programs/Therm%20Calc/NTCCalibrator/NTCcalculator.htm
Vishay also provides an on-line Steinhart-Hart calculator: https://www.vishay.com/en/thermistors/ntc-rt-calculator/
Hi, how do you connect a capacitor to the circuit above ? Will this make the thermistor more stable?
The rearranged formula for R2 seems to be incorrect. I believe the X (multiplication) should be a / (division).
Actually the rearranged formula works fine assuming R2 is the thermistor. My confusion was caused by the misalignment of the voltage divider formula, which measures voltage across R2, and the rearranged formula, which measures voltage across R1. Algebraicly you can’t rearrange the 1st formula to get the 2nd.
I think that if Vout = Vin* R2/(R1 + R2), then R2 = R1 * Vout / (Vin – Vout), not R2 = R1 * (Vin/Vout – 1) as indicated above. Had me confused for a while, but then I realised that R1 = R2 (Vin/Vout – 1). However, R1 is not the unknown. If we make R1 the unknown, then we end up with R1 = R2 * Vout / (Vin – Vout). I may actually build a circuit to see if this works. Thanks.
Alvin is correct. From the equation: Vout=Vin(R2/(R1+R2)), the R2 resistor is the one that connects to ground and R1 connects to 5V (or 3.3V). It appears you have the thermistor in the R1 position connected to 5V, but in your code comments you say R2 is the thermistor. If you swap the power and ground on the resistor circuit, then everything should work.
There is only one problem with this circuit. Recommended max impedance for the atmega 328 analog inputs is 10K. The circuit will work fine if using only one analog input, but the adc readings will not be stable if using another analog input.
Can you please elaborate? I don’t understand why there is a max impedance on the analog inputs. Shouldn’t they read any voltage value as it is? I was planning on using A0, A1, A2, and A3 for 4 different temperature sensors.
Hi. I’m working on a project where I have to avoid a certain temperature range. The range I’m avoiding is from 5 degree Celcius to 60 degree Celcius. What I really want is to add a condition that would display the words “Danger Zone” when the temperature is anywhere in between the said range. I was thinking along the lines of adding an if else statement with the following.
if
T> 5 || T<60;
lcd.print("Danger Zone");
else
Please can anyone correctly insert this line at the right spot and send me the whole code because I can't seem to make it work. I have to submit this project in the next two days so please if anyone is reading this, help a brother out. Thanks
will you send me the schematic circuit diagram for which shown in the video
so when i start code it says that the temp is -459.67 degrees F how do I fix this
HI,
I built the circuit as well and get the same result (-459.67 deg F). Were you able to determine what the problem is?
Thanks
Hi,
How do you setup a chain of thermistors?
Arduino has a limited numbar or ports.
hello, how much did this project cost you?
How can I get rid of the decimal so the LCD only displays a whole number like 76 F instead of 76.12 F?
Not sure if this works, but try Serial.print(T, 0);
I’ve got a 10K thermistor and 10K resistor hooked up, I am getting values but, for example, when I hold the thermistor the temp goes up but it goes slowly and maxes out at 83ish F. Do I need to change something because of my 10K components?
Your R1 value in sketch reads 10000, shouldn’t it be 100000 for a 100k resistor or am I missing something?
Thanks to all the folks who participated in this circuit review and discussion, it was very helpful. I purchased 100K thermistors from http://www.taydaelectronics.com and needed to do some tweaking of the co-efficiences. I was amazed at how little a change it took to change the temperature reading. The part number i purchased is the A-410. These thermistors are manufactured by Thinking Electronics from Taiwan . Here are the numbers I changed in the code: c1=0.7904710802e-3, c2=2.251846924e-4, c3=0.87060700625e-7
what are c1 c2 and c3 and how do u calculate them
https://www.thinksrs.com/downloads/programs/therm%20calc/ntccalibrator/ntccalculator.html
Hi! can i replace the thermistor with a peltier module?
hi my problem is that comes up with -999.00 for my temperature reading.How do you fix this?
fixed it:D
Is this method able to measure negative temperatures (like below 0 deg C), I failed to measure negative temperatures using LM35 and DHT 11.
What is the code if i add in 3 resistors instead of 1
I am having an issue with my thermistor outputting Fahrenheit as 198.73 at room temp… I cannot seem to figure out what the issue is but it is calculating F to C correctly. The values are just way too high.
Make sure you use a resistor that matches the resistance of the thermistor. Measure thermistor’s resistance with a multimeter and choose a resistor to match. I wired 10k and 12k resistors in series to get 12k to match the approx. 12k of the thermistor’s resistance.
The conversion from centigrade to F might be more readable if it were written F = C * (9/5) + 32. Maybe, I’m OCD about math.
hi,Why R1 is 1000? Should not be 100,000? you use 100k ntc and 100k resistor
Hi, i followed all the steps in the video, but im not getting any LCD output. Can anyone plz help?
Same issue bro…. may I know which resistors you used ??
Your explanation was good but you should atleast tell what resistor value are you using ??
I have built the exact same circuit but its not running (probably the resistors are issue)
Great article.. helped me a lot with my project.
although i get accurate readings from room temp. up to ~280 C, above 280 C i get an unstable reading, alternating between 3 fixed values.. 370.30 | 307.53 | -273.15 (same thing happens if i replace my thermi. with a 220 ohm resistor)..
so i assume the code can’t handle R2 values below a certain resistance..
any idea if i need to change c1, c2, c3 values ? or anything else..
(i’m using a HT-NTC100K that can handle up to 350 C)
thanks
Hello, I have a question is there was any way to display the voltage of the thermistor at any given time along with it’s temperature.
Just tried the temp sensor with LCD. Works great, but wondering how to get it to use only whole numbers. I don’t want to use up the extra 2 digits and the decimal point, how do I tell it to stick to whole numbers?
set the variable T as an int instead of a float.
Great compliments, this website is awesome! Everything is clearly explained, great thanks!
please can any one help me write a code of humidity sensor range between 80-85
Hay man tanks for the code it works but a slight problem when it is like cold or when i gave some cold air the temperature doesn’t comes below 29,28 it shows some Chinese,japanes languages on the display on the other when i heat the thermistor the temperature rises & displays 70,80,90 thats not a issue
So how do i display lower readings??
The equation derived for R2 looks wrong. Try plugging known variables into the voltage divider equation to get Vo, then plug these values back into the second equation and you’ll get a new (and therefor incorrect) R2 value. Instead, it should be R2 = (Vo/(Vi-Vo))*R1 = R2
what is c1,c2 and c3 and how do u calculate them
Hello,
the code works perfectly for arduino, but it does not gives me the goods values woth a ESP8266 :'(
Is it a formula problem?
Thanks
Has anyone worked out the Math for an ESP32? I built this with an Arduino UNO and it worked perfectly. Now I’m doing it on an ESP32 and the temperatures are running around 200 degrees. I like to keep my house warm in the winter but not quite that toasty. Can anyone shed any light on this??
Thanks!!
Hi,
I tried this project with an NTC 47D-15 thermistor. The resistance of this thermistor at room temp is about 45 ohm according to datasheet and my multimeter. I used a 220 ohm resistor. Also, I used the coefficients in your sketch. The temperatures returned by the sketch were in excess of 400! I’m sure I made some type of mistake although I feel confident about the wiring. I looked at the datasheet for my thermistor and didn’t find a listing of coefficients. Apparently, those can be determined by experimentation, but that sounds like a major project in itself. Would appreciate any suggestions.
Mike
Hello,
can anyone assist me? I am using a different thermistor and LCD screen than shown here. I have managed to modify the code to output the temp display in C on the LCD screen I am using, however, it is not accurate. I produced a 3-point calibration curve and have a linear trendline displaying the equation. Can I modify this code with my calibration equation?
My calibration equation is y=0.2194x-50.589
Attached is my code.
#include
int ThermistorPin = 0;
int Vo;
float R1 = 10000;
float logR2, R2, T;
float c1 = 1.009249522e-03, c2 = 2.378405444e-04, c3 = 2.019202697e-07;
// Attach the serial enabld LCD’s RX line to digital pin 11
SoftwareSerial LCD(10, 11); // Arduino SS_RX = pin 10 (unused), Arduino SS_TX = pin 11
void setup()
{
LCD.begin(9600); // set up serial port for 9600 baud
delay(500); // wait for display to boot up
}
void loop() {
Vo = analogRead(ThermistorPin);
R2 = R1 * (1023.0 / (float)Vo – 1.0);
logR2 = log(R2);
T = (1.0 / (c1 + c2*logR2 + c3*logR2*logR2*logR2));
T = T – 273.15;
// T = (T * 9.0)/ 5.0 + 32.0;
// move cursor to beginning of first line
LCD.write(254);
LCD.write(128);
LCD.print(“Temp = “);
// move cursor to
LCD.write(254);
LCD.write(133);
LCD.print(T);
// move cursor to
LCD.write(254);
LCD.write(139);
LCD.print(” C”);
delay(500);
LCD.write(” “);
delay(1000);
}
Look a bit higher up in the comments. Find the comment by Ronald and use the link to find your c-factors. Put those in place of the given ones and voila it works.
If your resistance rises as temperature increases you’ve got a ptc and it won’t work with this setup.
I do not understand your equation for the resistance with the y and x.
What did you calculate here and how?
The resistance/temp coordinates you use for the calculation should be as far apart as possible I.e. freezer boiling water and room temp.
Hi, thanks for this help, because I can now read the temperature of a 3d printer’s hotbed with my arduino !
Hello and thank you for this excellent tutorial.
I have a question about defining Vo as an integer. Will that make a difference in this command?
Vo = analogRead(ThermistorPin)
Wouldn’t it make more sense to define Vo as a float, or does it not matter?
Thank you!
-Marcy
Ah nevermind! Vo is an integer in the range of 0 to 1023, I forgot that part. Actual readings for our thermistor are 218 to 237, which are then converted to resistance R2. Got it.
-Marcy
really nice and cool post
Hello,
why you declare R1=10000 in program and in your circuit the resistor has value 100K Ohms?
Thanks in advance
I wired everything correctly, i double checked and everything was fine, but its showing negative stats, like “Temp = -32.47 F”. And its really low to, on my thermastat it says 75 F. How to fix this
I found this article a long time ago, and since I had a lot of thermistors (pack of 25 for some strange reason!) I decided to hard wire one together to just have around in case I wanted to use it for some reason. Well I found it today and was trying to figure out how the heck I had it wired up, did a search and took me back to this article. It made things quite easy and I have it up and running again. Still don’t know what the hell I am going to do with 25 thermistors, or even the one I built up on a strip board, but they are fun to tinker with. I have a niece who is a ghost hunter, and I think that was what I had in mind when I ordered the lot, I have built her several EDF meters from a simple one transistor to a very complicated Arduino based with 12 LED’s to indicate how strong the EDF field was. I even made her one that talked, but it scared the hell out of her hunting club on an outing to a haunted hotel in Deadwood, so she brought it back so I could part it out. They say a sudden drop in tempature is present when a spirit is in the room, that was the thought behind using several of these thermistors in, say a strip so you could measure the temp change in say one yard or even just a foot or so. Indeed, that was IT ! Think I will get back on that project now that I figured it out once more. Thanks a million for jogging my 70 year old mind back to ground level, it has been rather sluggish after sitting with my wonderful wife of 51 years as cancer took her from me, just 4 days after our 51st wedding anniversary. What do you get your wife for that occasion? Well I got her 6 red roses, 5 for the 50 and a pink one for the 51st. She loved it. I buried her with a gold plated rose in her hand, wearing the same dress she wore on that day in 1969 when we joined our souls for life.
Thank you Sir.
Can we use RTD Or Thermocouple in place of NTC?
Regards
An improvement regarding NTC temperature calculation if you use a 100k ohm resistor and a 100k ohm @25°C NTC in the voltage divider: you should change values with the follows:
float R1 = 100000; // 100k Ohm voltage divider resistor
float c1 = 6.66082410500E-004; // Steinhart-Hart C1
float c2 = 2.23928204100E-004; // Steinhart-Hart C2
float c3 = 7.19951882000E-008; // Steinhart-Hart C3
Other values can be calculated here:
https://www.thinksrs.com/downloads/programs/therm%20calc/ntccalibrator/ntccalculator.html
:-)
There is a wrong R2 equation. R2=R1*(Vout/(Vin-Vout)).
You have R2=R1*(Vin/Vout – 1).
Anyone know of a way to assign an IP address to access the temp reading? Ive seen many other codes that will add the wifi ability but they don’t show the proper temperature like this code does. I would love any help. THANKS!!!!
Hi, what if i have a 500 000 kilo ohm thermistor value.What resistor value should i use? Thank you
Hi there.
I just tried do make this circuit and everything works perfectly except one detail: the temperature is in fahrenheit but i need it in celsius. what do i have to change on the code for it to display temperature in celsius?
The second set of code given is an answer to this question
hello, i have some problem and I can´t deal with it. There is still just a one value on my lcd. Still -17,78°C (I have konverted it into this type of units). Can anyone help me please ?
Hi, can you check if your thermistor works with an ohmmeter ?
And if it’s fully linked to the motherboard ?
Otherwise the value can’t be read.
What value have you received before conversion ?
Works perfectly and i have a question,
I want to try it out and apply this on my fridge but i’m struggling with something i want to add.
I want to send a sginal do Shield SIM800F and send a TEMP HIGH SMS if the temperature is higher than 10Cº and another SMS TEMP HIGH if the freezer is higher than 13Cº.
Can you help me?
Hi guys, I am having a hard time following an equation conversion shown above. When converting myself, I get a different result for the final equation.
Here’s the starting equation:
Vo = Vi (R2 / (R1 + R2))
And here we go…
Vo / Vi = R2 / (R1 + R2)
Vi / Vo = (R1 + R2) / R2 = (R1 / R2) + 1
(Vi / Vo) – 1 = R1 / R2
R2 = R1 / ((Vi / Vo) – 1)
Note the only difference is that the multiplication of R1 with the rest in the solution shown in the above article, is now division. Am I forgetting how to do algebra with fractions, or is this a mistake from the author? It shows up in the code as well.
Can I get the circuit diagram