The DHT11 humidity and temperature sensor makes it really easy to add humidity and temperature data to your DIY electronics projects. It’s perfect for remote weather stations, home environmental control systems, and farm or garden monitoring systems.
In this tutorial, I’ll first go into a little background about humidity, then I’ll explain how the DHT11 measures humidity. After that, I’ll show you how to connect the DHT11 to an Arduino and give you some example code so you can use the DHT11 in your own projects.
Watch the video for this tutorial here:
Here are the ranges and accuracy of the DHT11:
- Humidity Range: 20-90% RH
- Humidity Accuracy: ±5% RH
- Temperature Range: 0-50 °C
- Temperature Accuracy: ±2% °C
- Operating Voltage: 3V to 5.5V
The DHT11 Datasheet:
What is Relative Humidity?
The DHT11 measures relative humidity. Relative humidity is the amount of water vapor in air vs. the saturation point of water vapor in air. At the saturation point, water vapor starts to condense and accumulate on surfaces forming dew.
The saturation point changes with air temperature. Cold air can hold less water vapor before it becomes saturated, and hot air can hold more water vapor before it becomes saturated.
The formula to calculate relative humidity is:
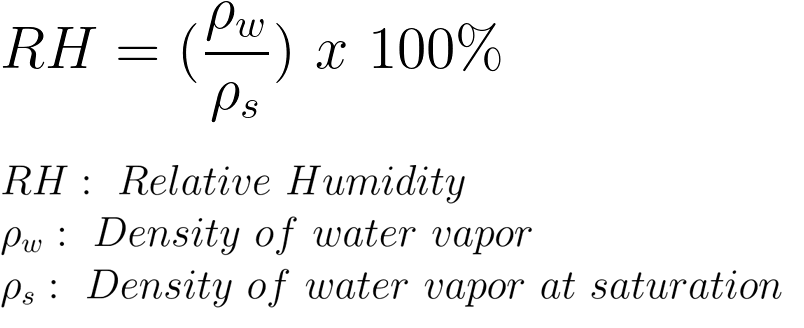
Relative humidity is expressed as a percentage. At 100% RH, condensation occurs, and at 0% RH, the air is completely dry.
How the DHT11 Measures Humidity and Temperature
The DHT11 detects water vapor by measuring the electrical resistance between two electrodes. The humidity sensing component is a moisture holding substrate with electrodes applied to the surface. When water vapor is absorbed by the substrate, ions are released by the substrate which increases the conductivity between the electrodes. The change in resistance between the two electrodes is proportional to the relative humidity. Higher relative humidity decreases the resistance between the electrodes, while lower relative humidity increases the resistance between the electrodes.
The DHT11 measures temperature with a surface mounted NTC temperature sensor (thermistor) built into the unit. To learn more about how thermistors work and how to use them on the Arduino, check out our Arduino Thermistor Temperature Sensor Tutorial.
With the plastic housing removed, you can see the electrodes applied to the substrate:
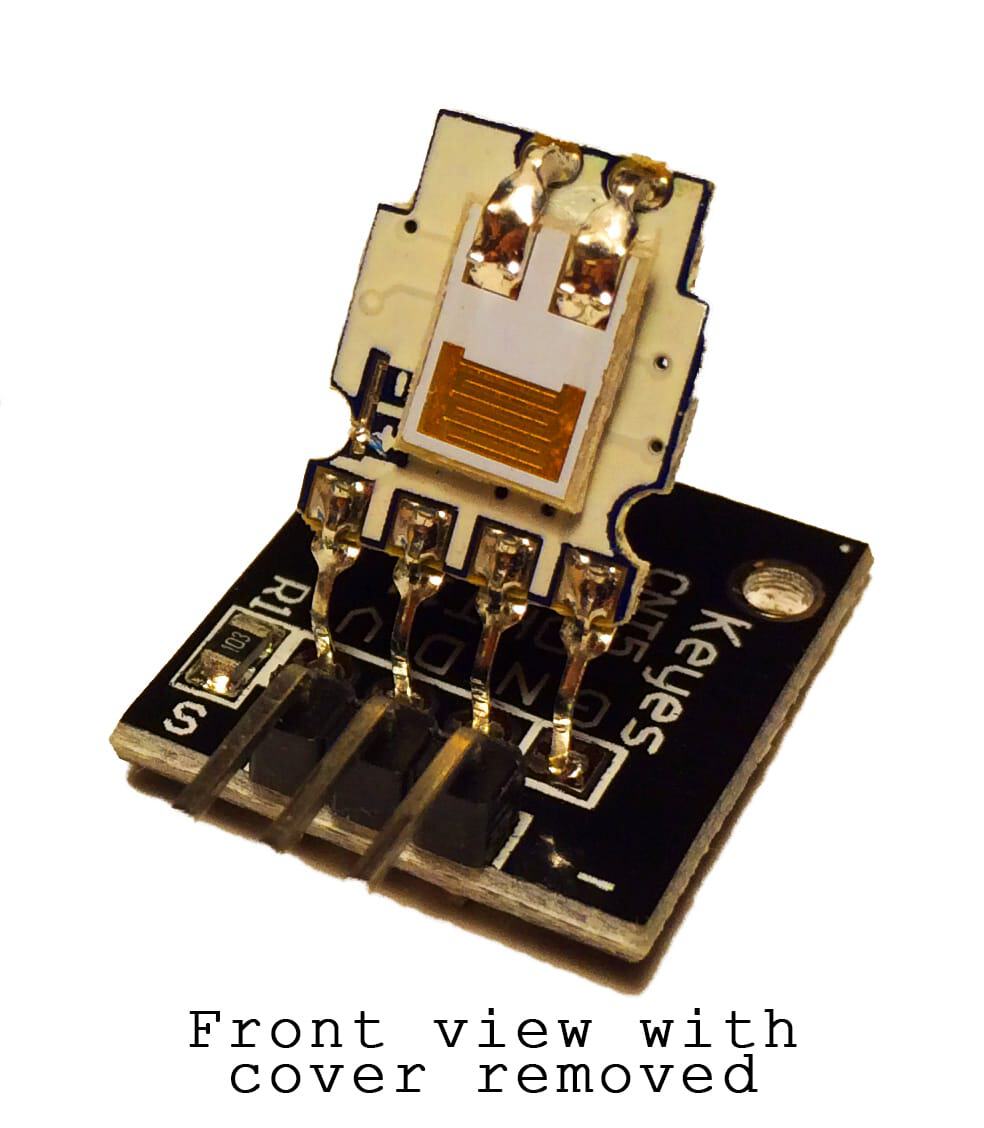
An IC mounted on the back of the unit converts the resistance measurement to relative humidity. It also stores the calibration coefficients, and controls the data signal transmission between the DHT11 and the Arduino:
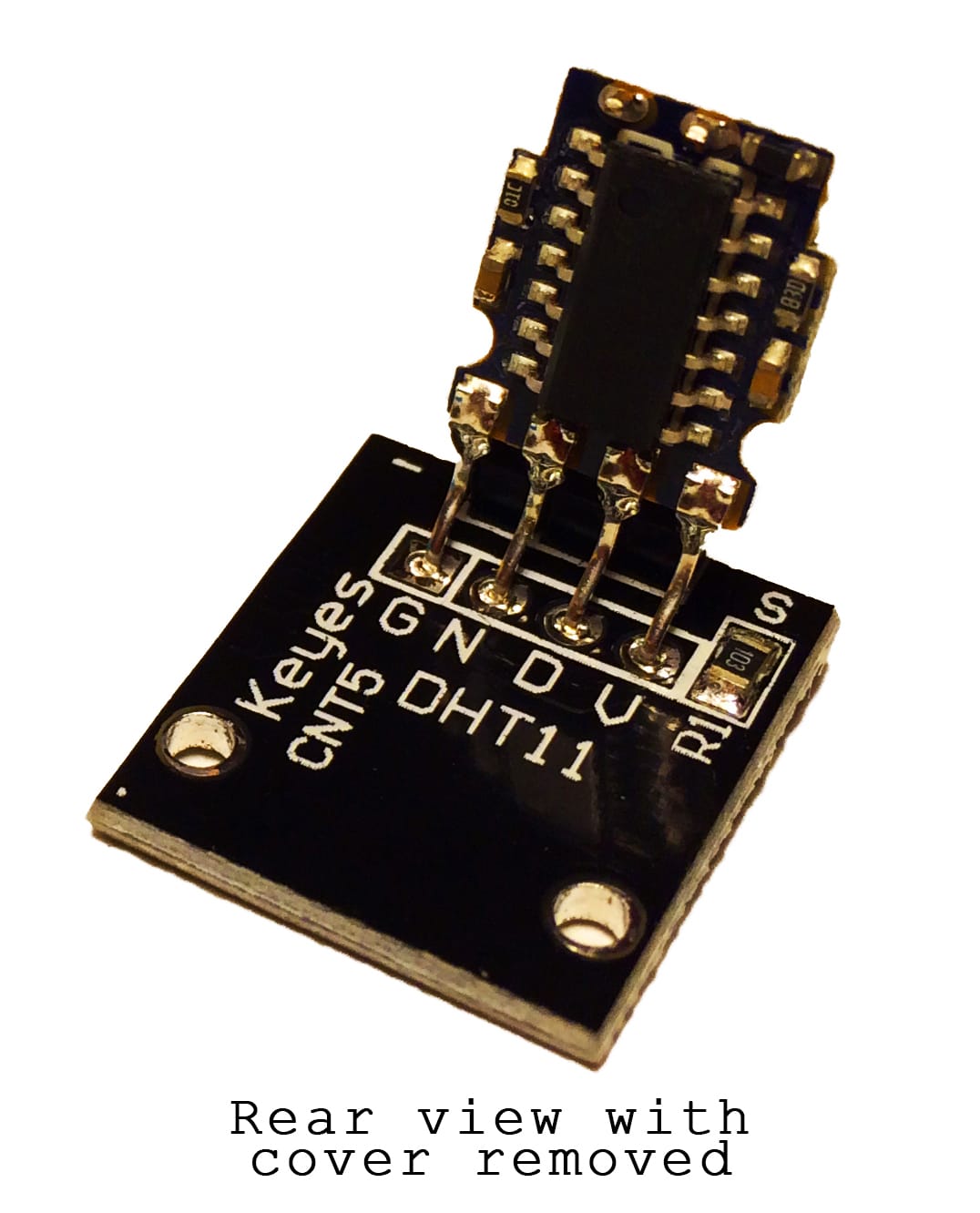
The DHT11 uses just one signal wire to transmit data to the Arduino. Power comes from separate 5V and ground wires. A 10K Ohm pull-up resistor is needed between the signal line and 5V line to make sure the signal level stays high by default (see the datasheet for more info).
There are two different versions of the DHT11 you might come across. One type has four pins, and the other type has three pins and is mounted to a small PCB. The PCB mounted version is nice because it includes a surface mounted 10K Ohm pull up resistor for the signal line. Here are the pin outs for both versions:
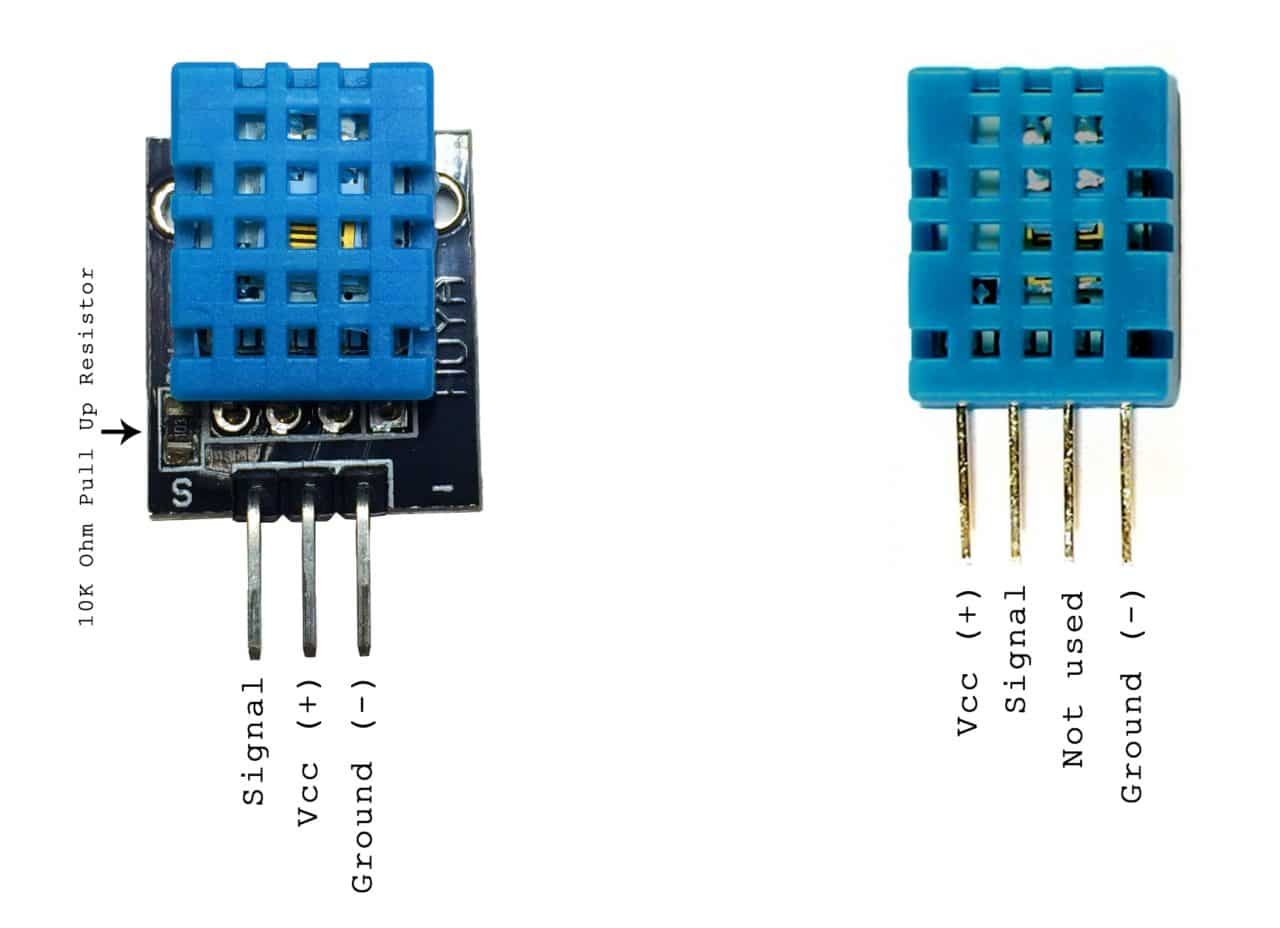
How to Set Up the DHT11 on an Arduino
Wiring the DHT11 to the Arduino is really easy, but the connections are different depending on which type you have.
Connecting a Three Pin DHT11:
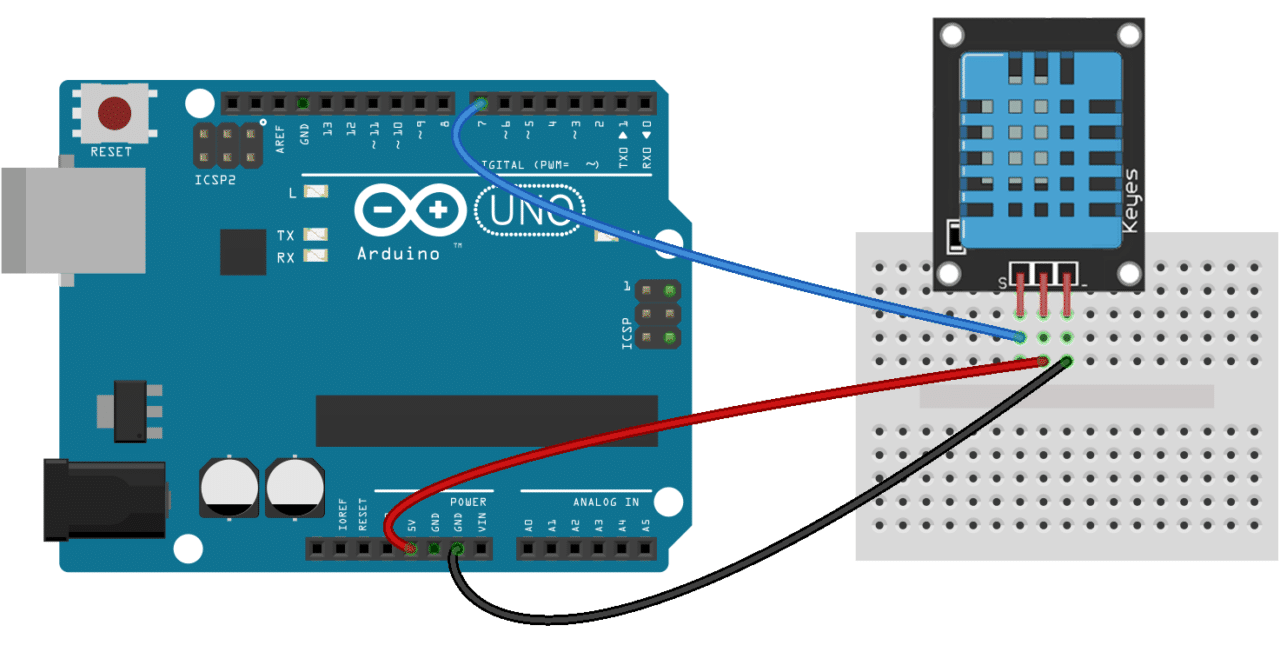
Connecting a Four Pin DHT11:
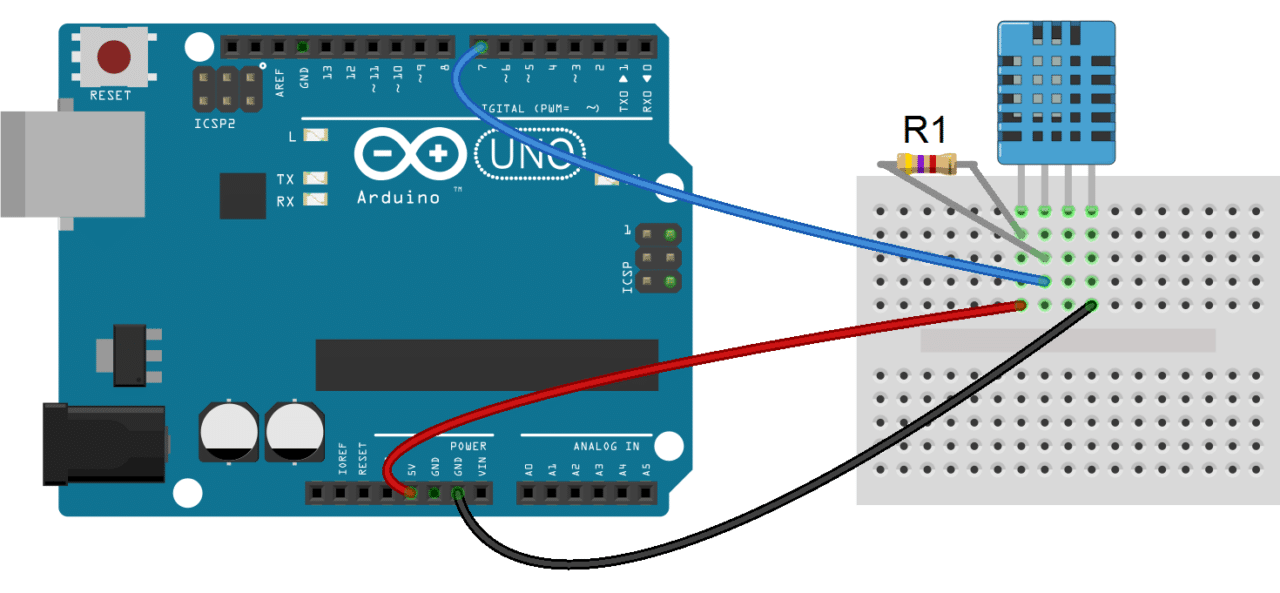
- R1: 10K Ohm pull up resistor
Display Humidity and Temperature on the Serial Monitor
Before you can use the DHT11 on the Arduino, you’ll need to install the DHTLib library. It has all the functions needed to get the humidity and temperature readings from the sensor. It’s easy to install, just download the DHTLib.zip file below and open up the Arduino IDE. Then go to Sketch>Include Library>Add .ZIP Library and select the DHTLib.zip file.
After it’s installed, upload this example program to the Arduino and open the serial monitor:
#include <dht.h>
dht DHT;
#define DHT11_PIN 7
void setup(){
Serial.begin(9600);
}
void loop(){
int chk = DHT.read11(DHT11_PIN);
Serial.print("Temperature = ");
Serial.println(DHT.temperature);
Serial.print("Humidity = ");
Serial.println(DHT.humidity);
delay(1000);
}
You should see the humidity and temperature readings displayed at one second intervals.
If you don’t want to use pin 7 for the data signal, you can change the pin number in line 5 where it says #define DHT11_PIN 7
.
Display Humidity and Temperature on an LCD
A nice way to display the humidity and temperature readings is on a 16X2 LCD. To do this, first follow our tutorial on How to Set Up an LCD Display on an Arduino, then upload this code to the Arduino:
#include <dht.h>
#include <LiquidCrystal.h>
LiquidCrystal lcd(12, 11, 5, 4, 3, 2);
dht DHT;
#define DHT11_PIN 7
void setup(){
lcd.begin(16, 2);
}
void loop(){
int chk = DHT.read11(DHT11_PIN);
lcd.setCursor(0,0);
lcd.print("Temp: ");
lcd.print(DHT.temperature);
lcd.print((char)223);
lcd.print("C");
lcd.setCursor(0,1);
lcd.print("Humidity: ");
lcd.print(DHT.humidity);
lcd.print("%");
delay(1000);
}
Using the Data in Other Programs
What if you don’t want to output the actual humidity and temperature readings, but need them to calculate or control other things? The code below is the bare minimum needed to initialize the sensor. You can add this to existing programs and use DHT.humidity
and DHT.temperature
as variables in any function.
#include <dht.h>
dht DHT;
#define DHT11_PIN 7
void setup(){
}
void loop(){
int chk = DHT.read11(DHT11_PIN);
delay(1000);
}
To see an example of using the DHT11 sensor outputs as variables in other functions, check out our article How to Set Up an Ultrasonic Range Finder on an Arduino, where we use the DHT.humidity
and DHT.temperature
variables in a formula that improves the accuracy of an ultrasonic range finder.
You can watch how to set up the DHT11 and see how it works in this video:
If you have any questions about how to set up the DHT11 humidity and temperature sensor on your Arduino, just leave a comment below and I will try to answer it… And if you like our tutorials, please subscribe! Also, feel free to share this if you know anyone else that might find it helpful!
Thank you
Hi.
It is discussed on the net you get different measurement results from DHT11.
The factory calibration are stored on the chip.
Can you make a recalibration?
Do you know hove to do it?
Regards.
Peter
Hi Peter, I haven’t tried re calibrating the factory values, but the datasheet has instructions on how to do it
how to connect dht11 sensor with raspberry pi 3????
We have a tutorial on that too: https://www.circuitbasics.com/how-to-set-up-the-dht11-humidity-sensor-on-the-raspberry-pi/
How would the code look to display in Fahrenheit rather than C
#include
#include // Include the Time library
dht DHT;
#define DHT11_PIN 7
void setup() {
Serial.begin(9600);
}
void loop() {
int chk = DHT.read11(DHT11_PIN);
// Convert Celsius to Fahrenheit
float temperatureCelsius = DHT.temperature;
float temperatureFahrenheit = (temperatureCelsius * 9/5) + 32;
Serial.print(“Temperature = “);
Serial.print(temperatureFahrenheit); // Output in Fahrenheit
Serial.println(” °F”);
Serial.print(“Humidity = “);
Serial.print(DHT.humidity);
Serial.println(“%”);
delay(12000);
}
Can anyone tell me how to activate/deactivate s pin depending on temp?
my display does not work. only back light is on
Mueed, do you have more information?
Do you have an I2C connected for instance?
Do you have a code for LCD i2c ?
I have a code that works for ic2
:
#include
#include
#include
LiquidCrystal_I2C lcd(0x27,16,2);
dht DHT;
#define DHT11_PIN 8
void setup(){
lcd.init();
lcd.backlight();
}
void loop()
{
int chk = DHT.read11(DHT11_PIN);
lcd.clear();
lcd.print(DHT.temperature);
lcd.print(“c “);
lcd.print(DHT.humidity);
lcd.print(“% “);
delay(2000);
}
how to connect esp8266 wifi module in arduino uno?
I have the dht11 reading and printing to lcd and serial monitor.I have the dht11 controlling two relays one for temp and one for humidity.When the relay turns on the dht11 stops sending readings and freezes and stops reading? Any way I can fix that ?thanks
Hi Kevin,
When the DHT11 triggers, do you have your code setting a different pin HI?
thank you
Hi,
Do you happen to have a schematic of the LCD display? If so then that would be helpful.
Thanks,
Zach
it shows -999 with both the temperature and humidity.
what do i do?
I got the same -999 readings alternating with good readings. I fixed it by adding a delay(1000); after the read command:
#include
dht DHT;
#define DHT11_PIN 7
void setup(){
Serial.begin(9600);
}
void loop()
{
int chk = DHT.read11(DHT11_PIN);
delay(1000);
Serial.print(“Temperature = “);
Serial.println(DHT.temperature);
delay(1000);
Serial.print(“Humidity = “);
Serial.println(DHT.humidity);
delay(1000);
}
Thanks for the info.
This didn’t do it for me, any other solutions?
Code looks like this:
#include
dht DHT;
#define DHT11_PIN 7
void setup(){
Serial.begin(9600);
}
void loop()
{
int chk = DHT.read11(DHT11_PIN);
delay(1000);
Serial.print(“Temperature = “);
Serial.println(DHT.temperature);
delay(1000);
Serial.print(“Humidity = “);
Serial.println(DHT.humidity);
delay(1000);
}
#include
#include
DHT dht(2,DHT11);
void setup(){
serial.begin(9600);
dht.begin();
}
void loop(){
float h=dht.readHumidity();
float tc=dht.readTemperature();
float tf=dht.readTemperature(true);
Serial.print(h);
Serial.print(“%HUMIDITY/t”);
Serial.print(tc);
Serial.print(“*c Temperature /t”);
Serial.print(tf);
Serial.print(“* f Temperature /t”);
Serial.printIn(“”);
}
I’m having the same issue with -999.00 readings for both temperature and humidity. Not sure how to fix it.
From what I’ve read from the datasheet it can’t be read from more than once every 2 seconds. Changing the delay to 2000 cleared the issue up right away for me
To get rid off the 999 , set in the code before reading the Dht11:
pinMode({signalpin nr}, LOW)
I changed the delay to 1500 and that fixed it.
I changed the delay to 1500 and that fixed it.
Hi , can i find python source code for this app and thanks
When I hook up my sensor de return “nan”, which mens “not a number”. Does this mean that the sensor is broken or … ?
ciaoo
no problem
Temperature sensor i have connected b y seeing the circuit diagram
what is the output of temperature sensor? and how to capture output of sensor?
The output is to the serial monitor, unless you have connected an LCD. The video will show you how to open the serial monitor if you don’t already know how to.
I love this so much!! THANK YOU!!!
A quick question tho, do you have a tutorial on how to connect this to a wireless transceiver?? also in theory could i connect more then one humidity detector to an arduino in order to detect humidity from more then one spot? Thank you again and i’ve subscribed!
Hi Jose, you can definitely connect more than one sensor to a single Arduino. You would basically duplicate the code, and have a separate pins read the data from each sensor. As for connecting them to a wireless tranceiver, I’m sure it’s possible, but you would probably need to use another microcontroller as a hub to transmit the data. I haven’t tried it yet though, so don’t take my word for it!
no you can’t
Is there a way to change it from Celsius to Fahrenheit
The DHT.h library will do it for you. float f = dht.readTemperature(true);
Look at the example sketch also.
int tempF = DHT.temperature * 9 / 5 + 32;
// you then println tempF instead of DHT.temperature
To convert from celsius to fahrenheit use the formula below.
T(°F) = T(°C) × 9/5 + 32
or
T(°F) = T(°C) × 1.8 + 32
Also here is the code if this would be easier.
int celtemp = DHT.temperature;
int fartemp = (celtemp*1.8)+32;
Serial.println(fartemp);
where do you put these lines I just copied the example for the temp sensor with lcd display
I am trying to print to lcd the temperature in Fahrenheit. I have seen other authors code and cant seem to make them work. My code is below.
@@@@@@@@@@@@@ My Code @@@@@@@@@@@@@@@@@@@@
#include
#include
LiquidCrystal lcd(3, 4, 5, 6, 7, 8);
#define dhtpin A0// the output pin of DH11
dht DHT;
void setup() {
Serial.begin(9600);
lcd.begin(16,2);
}
void loop() {
int val= DHT.read11(7);
int cel= DHT.temperature;
int humi=DHT.humidity;
int tempF = DHT.temperature * 9 / 5 + 32;
lcd.print(“Temperature: “);
lcd.print(true);// display the temperature
lcd.print((char)223);
lcd.print(cel);
lcd.setCursor(0, 1);
lcd.print(“humidity: “);
lcd.print(humi); // display the humidity
delay(1000);
lcd.clear();
}
Hello, I built my first arduino project (measuring the room temperature and humidity with the DHT11) during Christmas holidays. The readings of the values were shown on the screen of my laptop. The measured room temperature was correct, but the measured humidity was much too low (about 20%RH). What can be the reason for ithe low humidity? And how can the sensor (if needed) be recalibrated?
Looking forward to your answer.
Thanks in advance.
Based on my AprilAire Humidfier’s adjustment knob, the linear equation for the humidfier is
“HumidGoal = 0.5*(outside T(°F)) + 25” (using Excel’s ability to provide an equation on a graph)
So if it is -20°F, it is probably acceptable. Household humidity drops in the winter as your furnace runs.
Big thamks for uploading the video. it was really useful.how can i get program to interface different sensors on arudino and display sensor values??
I haven’t tried connecting multiple sensors, but it should be fairly easy. You would just duplicate the code and use a separate pin to read the data for each sensor
Hi.
It is discussed on the net you get different measurement results from DHT11.
The factory calibration are stored on the chip.
Can you make a recalibration?
Do you know hove to do it?
Regards.
Peter
The datasheet will tell you how to recalibrate
I inverted the signal and + connectors by error. May I have burned the sensor?
I have the same Keyes sensor as in this tutorial.
Thanks, Juan
Probably not, since the signal is at the same voltage as Vcc. If you swap the Vcc and signal pins, the output will just read -999.00 for temp and humidity.
sir i m still getting -999.oo as temp and humidity aftr swapping both ways
I’m getting – 999 value too… Any idea?
vcc is the left one, signal the middle one and ground is the round one, in case of a 3 pin DHT11. the diagram above is not right. i was getting the same problem here.
sir i m still getting -999.oo as temp and humidity aftr swapping both ways
hi guys.. i found the bug.. u need to pull up data/out/signal line with 10K to +ve line,
Please do chk with multimeter.. u should get 10K between these two lines.. (S & +)
IN MY CASE IN THE DHT-11 BOARD WRONG RESISTOR WAS SOLDERED, WITHOUT KNOWING TAT I HAD TRIED ALL STUFF, GIVEN 10K PULL UP ADDITIONALLY.. DIDN’T WORKED FINALLY TRACED THE RESISTANCE BETWEEN PINS IT WAS 5 OHMS.. THEN BACK TRACED & REMOVED TAT & PULLED UP WITH 10k SOLVED MY ISSUE.. GUESS U TOO HAVE THE SAME ISSUE.. JUST CHK OUT..
CHETHAN BR
Thanks Chetan! That did the trick for me as well! And a big 10Q to Circuit Basics in the first place for all these wonderful tutorials.
The diagram is correct for most three pin DHT11 modules. Depending on the manufacturer, the pins on the PCB might be different though. The pins should be labelled with S for signal and “-” or “GND” ground.
the diagram is correct. The right pin is marked with “-” the left pin is marked”s” the centre pin is vcc.
I have this unit working connected as shown. I had readings of 999.9 for about 1 minute until internal calibration completed. Following that the sensor is performing well.
even after 5 minutes the readings show 999. why?
I had the same issue and change line 26
delay(1000);
to
delay(1500);
This solved the issue and showed the measured results
This worked for me! (Mine was flashing between 999*C and the results.)
Thanks a bunch :D
Hi.
I had the same problem width -999 .
This is my first day with Arduino Uno + DHT11 + Breadboard.
My mistake was that i powered on the bottom left part of the Breadboard.
And put the sensor in the right slots.
Then i understood, that the breadboard has not 2 power circuits (top and bottom), but four (top left, top right, bottom left, bottom right). This is the thing which was never said on youtube)
hello
I would like to know what these three lines mean
int chk = DHT.read11(DHT11_PIN);
Serial.print(“Temperature = “);
Serial.println(DHT.temperature);
int chk = DHT.read11(DHT11_PIN); reads the signal pin of the DHT11 (pin 7) that is defined in #define DHT11_PIN 7.
Serial.print(“Temperature = “); simply prints “Temperature = ” to the serial monitor.
Serial.println(DHT.temperature); prints the actual temperature reading from the DHT11 to the serial monitor
thanks for the good work. mine kept dispalying -999.00c on both humidity and temperature. what could be the problem?
line 1 reads the integer value of the dht11 sensor pin
line 2 simply print the phrase temperature=
line 3 prints the value (temperature) given by the integer found in line 1. It is printed alongside the phrase in line 2
when i am verify this project its not complete the project
because this command (dht DHT;) ‘dht’ does not name a type
what i do please.
u have to download and save dht library in Arduno Libraries
I need full code of the sensor connected to LCD
See the section “Output Humidity and Temperature Readings to an LCD Display” on a desktop… If you are viewing it on mobile, the full code might not display. Hope this helps
Hey is it possible to run a serial monitor with the LCD screen hooked up as well?
Io l’ho fatto con questo codice che stampa a display le info e parallelamente lp fà anche sulla seriale, credo volessi questo:
#include
#include
dht11 DHT11;
#define DHT11_PIN 7
LiquidCrystal_I2C lcd(0x27,16,2);
void setup(){
Serial.begin(9600);
lcd.init(); // initialize the lcd
}
void loop()
{
int chk = DHT11.read(DHT11_PIN);
Serial.print(“Temperature = “);
Serial.println(DHT11.temperature);
Serial.print(“Humidity = “);
Serial.println(DHT11.humidity);
lcd.backlight();
lcd.setCursor(0,0);
lcd.print(“Temp. “);
lcd.print((char)223);
lcd.print(“C = “);
lcd.print(DHT11.temperature);
lcd.setCursor(0,1);
lcd.print(“Umidita’ % = “);
lcd.print(DHT11.humidity);
delay(1000);
}
Thank you, works really nice the DHT Lib
Arduino: 1.6.8 (Windows 7), Board: “Arduino/Genuino Uno”
C:\Users\dillu\Documents\Arduino\my\my.ino:1:17: fatal error: dht.h: No such file or directory
#include
^
compilation terminated.
exit status 1
Error compiling for board Arduino/Genuino Uno.
PPllzzz help me sir.
ok sir now i got it
It’s probably because you need to install the DHT library
nice
works well
I’ve tried several DH11s and all of them are outputting 0.00 for temperature and humidity (in serial monitor) any idea what could be going wrong?
It could be something with the wiring… Could you double check that everything is wired correctly? Have you used a different pin for the signal?
try cheeck the line
int chk = DHT11.read(DHT11_PIN);
worked well on me
can you connect with me please i need your help
emy_elbehiry@yahoo.com
Excellent ..good job..
It’s nice after looking this.
I need a small help.Can I use the humidity and temperature readings later for predicting a rainfall?
So that if rain fall is spotted by the sensor so that I could give an alert to the user by sending a message to his phone.
Can you guys help me in this. All I want is to design a circuit that could predict a rainfall or water and send a message to the user to his phone.Also keeping in mind about the humidity and temperature factors.
This helps me in saving the laboratory equipment by predicting the water or rainfall.
Please help me with this.
Thank you
You would probably be better off using a barometer like the BMP180 for that. A falling barometric pressure can predict possible rainfall.
exit status 1
Error compiling for board Arduino/Genuino Uno.
can you help me plzzz
Exit status 1 means that there is an error compiling your code. Check out this thread: http://forum.arduino.cc/index.php?topic=365466.0
Thank you
Will it still work if you don’t solder
Sure, I set it up on a breadboard.
Could you set up both the LCD display and the sensor on the same breadboard. (I’m very new to this…)
Yeah you can, as long as the breadboard is big enough. I have a 84 x 55mm board that fits an LCD and the sensor. It’s a little tight though
What is that Ic used to send data to the arduino ….give me a number of that ic
The datasheet only says that it’s an 8-bit microcontroller. The chip itself doesn’t have any markings on it either so I’m not sure
how do i make this control relays
Check out this article: https://www.circuitbasics.com/build-an-arduino-controlled-power-outlet/
At the end of the article I use a DHT11 to control a 5V relay…
I have made another code for the serial Print:
#include
dht DHT;
#define DHT11_PIN 7
void setup(){
Serial.begin(9600);
delay(500);//Delay to let system boot
Serial.println(“DHT11 Humidity & temperature Sensor\n\n”);
delay(1000);//Wait before accessing Sensor
}//end “setup()”
void loop(){
//Start of Program
int chk = DHT.read11(DHT11_PIN);
Serial.print(“Current Humidity = “);
Serial.print(DHT.humidity);
Serial.print(“% “);
Serial.print(“Current Temperature = “);
Serial.print(DHT.temperature);
Serial.println(“C “);
delay(1000);//Wait 1 seconds before accessing sensor again.
//Fastest should be once every two seconds.
}// end loop()
Here is some simple code to output the DHT11 to a 16×2 LCD (with built in controller)
Outputs Fahrenheit, Celsius & Humidity:
Make sure you have the 3 libraries noted #include
// ***** Works for temp and humid display on LCD I2C 7/3/2016 *****
// Shows Fahrenheit, Celsius & Humidity
#include //library from …malpartida
#include
#include
//For LCD display 2 rows x 16 Characters
//For LCD with built in controller
//LCD pin SDA to Arduino Analog pin A4
//LCD pin SCL to Arduino Analog pin A5
//LCD power is 5V
//DHT11 Temp and humidity sensor in Celsius
//Signal wire of DHT11 to Arduino Digital pin 8
//DHT11 sensor power is 5V (middle pin on sensor)
LiquidCrystal_I2C lcd(0x3F, 2, 1, 0, 4, 5, 6, 7, 3, POSITIVE);
dht DHT;
/*—–( Declare Constants, Pin Numbers )—–*/
#define DHT11_PIN 8 //DHT11 Signal wire to pin 8
void setup()
{
lcd.begin(16,2); //16 by 2 character display
}
void loop()
{
delay(1000); //wait a sec (recommended for DHT11)
int chk = DHT.read11(DHT11_PIN);
switch (chk)
lcd.clear(); //Clears any previous message on LCD
//Print temp on line 1 (use 0 to indicate line 1)
//(0,0) indicates (Character position from left, Row 0=1 1=2)
lcd.setCursor(0,0); //next print line shows on LCD line 1
lcd.print(“Temp= “);
lcd.print(DHT.temperature, 0);
lcd.print(” C “);
lcd.print(DHT.temperature * 1.8 + 32, 0); //Fahrenheit conversion
lcd.print(” F “);
//Print Humidity on line 2 (use 1 to indicate line 2)
//(0,1) indicates (Character position from left, Row 0=1 1=2)
lcd.setCursor(0,1); //next print line shows on LCD line 2
lcd.print(“Humidity = “);
lcd.print(DHT.humidity, 0);
lcd.print(” %”);
delay(15000); //Shows data for 15 sec then refreshes screen with next line
lcd.clear(); //Clears any previous message on LCD
}
For some reason the 3 libraries to include were deleted from the code above.
Here they are:
#include
#include
#include
they keep getting blocked:
LiquidCrystal_I2C.h
dht.h
Wire.h
If i want to mix lcd,dht 11 and heater for use arduino?can you description program?
It sounds like you want to control the heater with the DHT11 and have the readings output to an LCD too… You can use the DHT11 to control the signal to a 5V relay, similar to what’s done in this article: https://www.circuitbasics.com/build-an-arduino-controlled-power-outlet/
Then you just need to add the code to initialize the LCD, include the LiquidCrystal library, and change the “serialprint()” functions to “lcd.print(). We have another article on setting up an LCD on the Arduino if you need help with it: https://www.circuitbasics.com/how-to-set-up-an-lcd-display-on-an-arduino/
hi. i am currently working on a project with arduino, lcd, dht11 sensor, and relays.
i didnt have any trouble interfacing the arduino, lcd and the dht11 sensor and my codes were quite right since when i run it, nothing’s odd in the output. but when i connect the relay,in which an ac device is connected, as an output that turns on after a couple of minutes, the temperature and humidity dislayed on the lcd becomes odd, like chinese and numbers, after some time. i checked my codes but i cant figure out whats wrong with it.
i hope you can help me out with this.
thank you…
Could you post the code here?
please help me.. i won’t get Alarm temperature and humidity..and show in lcd display 16×2.. and changeing temperature, humidity alarm set point HOW IS DO… PLEASE HELP ME.
Nice video but a bit hard to follow the lcd steps for a NOOB like me because of the angle of the camera.
Sorry about that… Thanks for the input! I’ll try to make it easier to see in future videos
Very nice! It’s very nice! Big thank you for the article, my friend! May you prosper and live long!
Thank you very much!
it is very nice project,i tried n working satisfactory.
So curiously, I had already downloaded and installed the latest version of DHTLib (v0.1.21) versus the older version (v0.1.14) that is provided here. And I kept getting 0.00 values for the temp and humidity readings as Alex reported on April 20, 2016 in a posting above. I scratched my head for a while until I remembered I had the newer version of the library installed. So I removed that, installed the older v0.1.14 version, and bam, lo and behold, I started getting real values back. So this may be the same problem that Alex had, too.
I’ve looked at the brief changelog history in dht.cpp file, and I’m seeing no obvious reason that might allow v0.1.14 to work, but not the newer v0.1.21. Anyone have thoughts about this?
Pretty informative. It’s good idea for projects. I am thinking of building my own weather unit soon. I think I can use some help from this. Thanks for sharing this!
Any comments about DHTLib v0.1.14 vs. v0.1.21, and why this simple Arduino sketch works in the former, but not the latter? The brief history in the cpp file header for v0.1.21 looks like it took care of a few issues so my first instinct is to use that, but again, it results in all zero readings. Anyway, if no comments, well, I’ll have to take a look through the diffs between the two versions to see what might be causing the issue.
That’s interesting, please post your findings if you see anything!
Thanks for the useful post.
I followed the instructions. Sensor connection is fine and have uploaded the code successfully.
But I am getting the output as -999. Can you please tell me the possible reason why I am facing this problem. Does it mean the sensor is faulty?
It is a DHTLIB_ERROR_TIMEOUT as I checked the value of chk in the code. Please help
hello…i’m also have the same problem…could u help me…
vcc is the left one, signal the middle one and ground is the right one, in case of a 3 pin DHT11. the diagram above is not right. i was getting the same problem here.
The diagram is correct, but your particular DHT11 could have a different pinout depending on the manufacturer. The DHT11 I used is from Keyes, what type do you have?
Are you using the four pin DHT11? If so you’ll need to put a 10K Ohm resistor between the Signal line and Vcc. I just added another diagram to the post to make it a bit clearer. That may be causing your issue.
nice #DIY replacement for Sling Psychrometer and chart https://t.co/Hnt5797GZr
is there a aerial view of how everything goes in?
Thanks a lot, may you please help me out, I am using a Mega 2560 with a DHT11 sensor, my problem is that both temperature and humidity reading is just being reed as 0.00 and they are not changing. What might i be doing wrongly, I have even tried the code that accompanies these tutorials
Which DHT library did you install? There is an issue with version 0.1.21. The download in the post above is version 0.1.14, which works fine.
This seems like a really simple setup, but I’ve been having a lot of trouble setting this up. Have there been changes to this library? I have downloaded it, but arduino still refuses to recognize dht or any of the related functions, like temperature/humidity. It had a lot of trouble with line 3, dht DHT;. Any advice?
Thanks for this great resource!
Yes, the library was updated recently (v. 0.1.21) and doesn’t seem to work. If you download the zip file I put in the post, it should work. It’s the older version 0.1.14.
Hi, you mentioned you added a piece of code to show the “degree” symbol,” lcd.print((char)223)”, can you tell me if the number 223 is from the ASCII table.
I have looked at the ASCII table and the number for the degree symbol is 248.
Can you please advise.
Many thanks in advance.
I get this error
This report would have more information with
“Show verbose output during compilation”
enabled in File > Preferences.
Arduino: 1.0.6 (Windows NT (unknown)), Board: “Arduino Uno”
sketch_sep16a:3: error: ‘dht’ does not name a type
sketch_sep16a.ino: In function ‘void loop()’:
sketch_sep16a:13: error: ‘DHT’ was not declared in this scope
can u give the codes for the serial display cause this code works only for the parallel display
and sir thank you you are very helpful and its very easily to understand :)
hai
I followed the instructions. Sensor connection is fine and have uploaded the code successfully.
But I am getting the output as -999. Can you please tell me the possible reason why I am facing this problem. Does it mean the sensor is faulty?
vcc is the left one, signal the middle one and ground is the right one, in case of a 3 pin DHT11. the diagram above is not right. i was getting the same problem here.
hello.
er..could u pliz explain how to set up the code so the temperature sensor,soil moistue sensor and humidity sensor can workout?im new in arduino..:’)
can this be used on a rpi?
Yes it can, actually we have another tutorial on how to set it up on the Raspberry Pi: https://www.circuitbasics.com/how-to-set-up-the-dht11-humidity-sensor-on-the-raspberry-pi/
i am doing fire alarm system using dht and lcd and GSM sim800l how can i make argument to send message from gsm if the sensor reading is higher that the set temp and how to declare it thanks for your response
hi! i’m kachi
After uploading a code my dht-11 keeps reading zero ‘0’ for both humidity and temperature as the output on my serial monitor. please what could be the problem?
i got a similar problem. getting output as -999.00 for both humidity and temperature.please help!!
getting output as -999.00 for both humidity and temperature.please help!!
Hi Kachi, I believe this is a problem with an updated version of the DHTLib library (version 0.1.21). If you download the library from this post (it’s version 0.1.14) instead of from github it should work.
i get this error while i upload the program
avrdude verification error first mismatch at byte 0x0000
plzz help
I am new in this game with very limited knowledge ,I found your detailed instructions very informative and accurate .
I am very happy to inform you that I fixed successfully the temp and humidity project with LCD display. I would like to subscribe but cannot find the link.Many thanks
gesto
Do you know how to average the readings from DHT11 using Ardunio Uno? thanks.
how can i output the average readings from DHT11 using Arduino uno
what is the values of resistors??
hello, can i know how to stop the sensor from reading? for example i only want it to read 10 value then stop. can anyone teach me?
You can do it in using for loop method. You should replace all the Serial.print lines to the “void setup()” function.
hello, I used Wemos D1 wifi based ESP8266 and dht 11 but there’s wrong in the code of cpp and .h i dont know what to do..
Arduino: 1.6.11 (Windows 8.1), Board: “WeMos D1(Retired), 80 MHz, 115200, 4M (3M SPIFFS)”
Build options changed, rebuilding all
In file included from C:\Users\mhine\Documents\Arduino\libraries\DHTLib\dht.h:18:0,
from C:\Users\mhine\Documents\Arduino\libraries\DHTLib\dht.cpp:30:
C:\Users\mhine\Documents\Arduino\libraries\DHTLib\dht.cpp: In member function ‘int dht::_readSensor(uint8_t, uint8_t)’:
C:\Users\mhine\AppData\Local\Arduino15\packages\esp8266\hardware\esp8266\2.2.0\cores\esp8266/Arduino.h:227:63: error: cannot convert ‘volatile uint32_t* {aka volatile unsigned int*}’ to ‘volatile uint8_t* {aka volatile unsigned char*}’ in initialization
#define portInputRegister(port) ((volatile uint32_t*) &GPI)
^
C:\Users\mhine\Documents\Arduino\libraries\DHTLib\dht.cpp:116:29: note: in expansion of macro ‘portInputRegister’
volatile uint8_t *PIR = portInputRegister(port);
^
exit status 1
Error compiling for board WeMos D1(Retired).
This report would have more information with
“Show verbose output during compilation”
option enabled in File -> Preferences.
Hi. I have the same issue with the same board. Did you get it to succeed in the end? I would be interested, but I feel that it may be a compatibility issue with a 3rd-party board. I have tried the exact code with other Arduinos that I have and it works just fine.
I get this same error when I try to use the Arduino 101 instead of the Uno. I think the library doesn’t support the board. I would try finding a different DHT library, there are several others out there.
Please can someone help me with a simulation circuit that will show the response graphs of dht11 for temperature and humidity
Problem with -999.0 also here… I don’t konw what to do…
My bad. I forgot to connect vcc wire to sensor :D
I got approx 1 of 5 reading that end with “Data not good, skip” Is it a sensor issue ??
Hi,
I Have issues with the Arduino recognizing the file dht.h. Was told no such file exist, meanwhile I have uploaded the zip file into the Arduino IDE, which showed in the file directory.
How do I solve this?
Did you use the library in the zip file from the post, or did you download it from the Arduino.cc page? Version 0.1.21 has some issues and doesn’t appear to work. The zip file in the post is version 0.1.14, and it does work. Also, are you using the Uno, or another board? I couldn’t get the library to work on my Arduino 101…
why this happening
No such file or directory
compilation terminated. sir
In this language, does declaring an object variable (as in “dht DHT;”) automatically instantiate it? I am more used to other languages that would need to follow the declaration with something along the lines of “DHT = new dht(params, for, constructor);” Does this normally go without saying in C++, or is this something the Arduino environment automatically adds at the preprocessing** stage?
**: If not “preprocessing,” then whatever else Arduino parlance calls the process of converting/expanding the “Processing” (??) or “Wiring” (???) code into standard C/C++ ????
should i connect pin 7 of arduino uno?
PD7 OR PB7
sir i m still getting -999.oo as temp and humidity aftr swapping both ways
DId you fix this? I am getting -999 for one second, then the correct readings for another second, flashing between the two.
i have the same, but i dont know how repair
NOW getting output as 0.00 for both humidity and temperature.please help!!
Try using the library in the zip file from the post (version 0.1.14). It sounds like you’re using the newer version (0.1.21) that is having some problems…
where should i 11 get dht11 library version 0.1.14?
pls reply..
thanks
Need Circuit Diagram of Arduino connecting with LCD
ASAP
THANKS
Check this article: https://www.circuitbasics.com/how-to-set-up-an-lcd-display-on-an-arduino/
ciao
I AM GETTING ERROR WHILE COMPILING THE PROGRA CODE
“avrdude: stk500_getsync(): not in sync: resp=0x00”
Hi, Is there a way to send alerts to an email address if the temperature and humidity is above a certain value?
Hi there,
I had en error when I run the first sketch.
Arduino: 1.8.0 (Mac OS X), Board: “Arduino/Genuino Uno”
humidity:22: error: redefinition of ‘dht DHT’
dht DHT;
^
/Users/anna/Documents/Arduino/humidity/humidity.ino:3:5: note: ‘dht DHT’ previously declared here
dht DHT;
^
/Users/anna/Documents/Arduino/humidity/humidity.ino: In function ‘void setup()’:
humidity:26: error: redefinition of ‘void setup()’
void setup(){
^
/Users/anna/Documents/Arduino/humidity/humidity.ino:7:6: note: ‘void setup()’ previously defined here
void setup(){
^
/Users/anna/Documents/Arduino/humidity/humidity.ino: In function ‘void loop()’:
humidity:30: error: redefinition of ‘void loop()’
void loop()
^
/Users/anna/Documents/Arduino/humidity/humidity.ino:11:6: note: ‘void loop()’ previously defined here
void loop()
^
exit status 1
redefinition of ‘dht DHT’
This report would have more information with
“Show verbose output during compilation”
option enabled in File -> Preferences.
What can I do?
Thanks!
bro i need some help .i want code for uploading the values read by sensor to web server.
Can someone help me to burn a light if the DHT11 detect something?
Would be great to see similar articles featuring MicroPython ?
Excellent article! I have a DHT11 on order and will refer to this when I test it out. Thank you so much. Oh.. and have followed on Twitter too!
the area of land it measures? approximately
did you find out? I am need this information too :(
I have programmed sensor DHT 11 using microcontroller, but i have not tried program this sensor using Arduino
sir please can you say me why it is responding as board COM1 unvailable
hey can you pls help me how to use rf module with the above project. i am using two arduino uno, DHT11, LCD, RF transmitter and receiver. please can u give me a code to display temperature and humidity on the receiver side lcd…
how about the new coding if i add another sensor which is smoke sensor (mq-2). Could you please show me the coding
why is the pull-up resistor needed?
let me konw if i understand it correctly:
the logic level on the data pin is HIGH, and that means the arduino and the dht are not communicating.
to start the cummunication the ardduino will give LOW to the data line,after the dht finished the transmition of data,the line will return to HIGH,IS THAT CORRECT???
why do you need the pull-up resistor?
let me know if i understant it correctly:
when thre is no communication between the arduino and the dht,the data line is HIGH
to star the communication the arduino will put LOW is the data line,after the transmiting is over the kine will return to HIGH
IS THAT CORRECT???
Is there any way to change it from Celsius to Fahrenheit?
#include
#include
LiquidCrystal lcd(12, 11, 5, 4, 3, 2);
dht DHT;
#define DHT11_PIN 7
void setup(){
lcd.begin(16, 2);
}
void loop()
{
delay(1000); //wait a sec (recommended for DHT11)
int chk = DHT.read11(DHT11_PIN);
switch (chk)
lcd.clear(); //Clears any previous message on LCD
lcd.setCursor(0,0);
lcd.print(“Temp: “);
//lcd.print(DHT.temperature);
//lcd.print(“C”);
lcd.print(DHT.temperature * 1.8 + 32); //Fahrenheit conversion
lcd.print((char)223);
lcd.print(“F”);
lcd.setCursor(0,1);
lcd.print(“Humidity: “);
lcd.print(DHT.humidity);
lcd.print(“%”);
delay(15000); //Shows data for 15 sec then refreshes screen with next line
lcd.clear(); //Clears any previous message on LCD
}
Libraries: dht.h, LiquidCrystal.h
Thanks for the mod, skyfox66. Being in America among the holdouts, I am of course still using degrees F. After days of struggling and searching I finally got this combination of parts and code to work right. (After I found this website).
The code does not compile and givesxan error here: int chk = DHT.read22(DHT22_PIN);
CBasics_DHT_LCD_Modded:19:18: error: expected primary-expression before ‘.’ token
int chk = DHT.read22(DHT22_PIN);
How can storage them date on sd-card?
Hello.. can i know how to programme dht11 with basic stamp 2 ?
I connected the LCD and the DHT11 and copied and pasted the code. It uploaded and then I look at my LCD and all I see are white boxes on the top of the display. Can anyone help me?
I also need to know how to change from celsius to farehneit. As an older generation person, i still don’t always think metric!
I copied this exactly and got it to display temperature and humidity, but it flashes -999 for temp and -999 for humidity every other second. For example, it will display correct readings for one second, then the -999 for both readings the next second.. Flashing between the two. Any ideas why it might be doing this. I have been playing with the code, rechecking pins, etc, but I cant seem to pinpoint the problem. Any input is appreciated.
Hi LM
Sorry, no answer, but I am having the same problem. My board is a Chinese clone of a MEGA2560 that came with a 20$ kit.
How to connect 3 form dht11 together with arduino?
Excuse me, what’s the work of line 3 ? “dht DHT;”
Is it possible to connect line 3 with dht11?
How to fix this?
Temperature = -999.00
Humidity = -999.00
Temperature = 23.00
Humidity = 27.00
repeating…..
Hi,
I solved the “999” problem by changing the “delay” from 1000 to 2000.
Currently, I am looking for the reason but I think that has something with the reading resolution of the sensor…
BR
Miki
the reason is:
// Make sure we don’t poll the sensor too often
// – Max sample rate DHT11 is 1 Hz (duty cicle 1000 ms)
// – Max sample rate DHT22 is 0.5 Hz (duty cicle 2000 ms)
I’ve managed to pool without -999.00 using 1200ms delay.
See more details at the following link:
http://tsuts.tskoli.is/hopar/ROB2B_H4/arduino-DHT-master/DHT.cpp
Hi,
I copy the code and the library, but cannot seem to work. Please help. Below are the error.
Arduino: 1.6.6 (Windows 10), Board: “Arduino/Genuino Uno”
WARNING: Spurious .github folder in ‘DHT sensor library’ library
Multiple libraries were found for “dht.h”
Used: C:\Users\Teddy\Documents\Arduino\libraries\DHTLib
Not used: C:\Program Files (x86)\Arduino\libraries\DHTLib
Sketch uses 4,714 bytes (14%) of program storage space. Maximum is 32,256 bytes.
Global variables use 98 bytes (4%) of dynamic memory, leaving 1,950 bytes for local variables. Maximum is 2,048 bytes.
avrdude: stk500_recv(): programmer is not responding
avrdude: stk500_getsync() attempt 1 of 10: not in sync: resp=0xb1
avrdude: stk500_recv(): programmer is not responding
avrdude: stk500_getsync() attempt 2 of 10: not in sync: resp=0xb1
avrdude: stk500_recv(): programmer is not responding
avrdude: stk500_getsync() attempt 3 of 10: not in sync: resp=0xb1
avrdude: stk500_recv(): programmer is not responding
avrdude: stk500_getsync() attempt 4 of 10: not in sync: resp=0xb1
avrdude: stk500_recv(): programmer is not responding
avrdude: stk500_getsync() attempt 5 of 10: not in sync: resp=0xb1
avrdude: stk500_recv(): programmer is not responding
avrdude: stk500_getsync() attempt 6 of 10: not in sync: resp=0xb1
avrdude: stk500_recv(): programmer is not responding
avrdude: stk500_getsync() attempt 7 of 10: not in sync: resp=0xb1
avrdude: stk500_recv(): programmer is not responding
avrdude: stk500_getsync() attempt 8 of 10: not in sync: resp=0xb1
avrdude: stk500_recv(): programmer is not responding
avrdude: stk500_getsync() attempt 9 of 10: not in sync: resp=0xb1
avrdude: stk500_recv(): programmer is not responding
avrdude: stk500_getsync() attempt 10 of 10: not in sync: resp=0xb1
Problem uploading to board. See http://www.arduino.cc/en/Guide/Troubleshooting#upload for suggestions.
WARNING: Spurious .github folder in ‘DHT sensor library’ library
WARNING: Spurious .github folder in ‘DHT sensor library’ library
This report would have more information with
“Show verbose output during compilation”
enabled in File > Preferences.
Regards,
Teddy
Where should I add a float line to get the temp and humidity to read out to 2 decimal places?
What should the line of code be… float…
hello, i need some help, i want code for, if i m sending message from mobile (e.g. ABC) to arduino via gsm module then the values of temperature and humidity receiving specific number
When I try to compile the sketch, I get the following error messages:
/Resources/Java/libraries/DHTLib/dht.cpp: In member function ‘int dht::_readSensor(uint8_t, uint8_t)’:
/Resources/Java/libraries/DHTLib/dht.cpp:114: error: ‘digitalPinToBitMask’ was not declared in this scope
/Resources/Java/libraries/DHTLib/dht.cpp:115: error: ‘digitalPinToPort’ was not declared in this scope
/Resources/Java/libraries/DHTLib/dht.cpp:116: error: ‘portInputRegister’ was not declared in this scope
Any ideas on how to correct this?
Thanks
Why does my LCD display switch back a forth between 999.000 and the correct measurement?
Hello,
May I ask why is there a decimal point but the numbers after it are always .00?
Also, I get that every other temp and humidity is -999 if I check at a rate of 1 sec, why is that so and how may I fix it?
Thanks in advance,
Ethan
Ethan I can’t help with the .00, But as for the -999 I just changed the delay to 2000 and it seem to take care of it.
Roger
But if I change the rate to 2000 ms it check well… slower.
Can’t I make it refresh normally but not do that?
Ethan
Hi, is it possible to control a servo motor using the DHT11 humidity sensor?
when i try to verify the code, I get an error saying ”
#include
^
compilation terminated.
exit status 1
Error compiling for board Arduino/Genuino Uno.”
what do i do?
p.s. i’m a complete noob with no C coding experience.
sorry, the full error message is:
fatal error: dht.h: No such file or directory
#include
^
compilation terminated.
exit status 1
Error compiling for board Arduino/Genuino Uno.
You have to upload the dth.h.zip library through Sketch tab.
i have the same problem! plz help!!!!
My output in the Serial Monitor reads the correct temp and hum but then gives a -999 for both values, then back to real values and so on.
Temperature = 21.00
Humidity = 60.00
Temperature = -999.00
Humidity = -999.00
Temperature = 21.00
Humidity = 60.00
Temperature = -999.00
Humidity = -999.00
Temperature = 21.00
Humidity = 60.00
Temperature = -999.00
Humidity = -999.00
Temperature = 21.00
Humidity = 60.00
Temperature = -999.00
Humidity = -999.00
Is this a problem with the sensor?
No, use same code but change delay to 2000:
#include
dht DHT;
#define DHT11_PIN 7
void setup(){
Serial.begin(9600);
}
void loop()
{
int chk = DHT.read11(DHT11_PIN);
Serial.print(“Temperature = “);
Serial.println(DHT.temperature);
Serial.print(“Humidity = “);
Serial.println(DHT.humidity);
delay(2000);
}
hi i would like to know hot to put the code for an lcd displa with an I2C thank you !
i used your library & sketch in my arduino
its output
Temperature = -999.00
Humidity = -999.00
Temperature = -999.00
Humidity = -999.00
Temperature = -999.00
Humidity = -999.00
Temperature = -999.00
Humidity = -999.00
Temperature = -999.00
how do i correct this plz help me
How far can the dht11 be located away from the board ? Can it have a long cable?
I was Googling around for content about weather station this morning, when I came across your excellent resource page.
I just wanted to say that your page helped me a ton.
It’s funny: I recently published a guide on weather station guide last month.
Here it is in case you’d like to check it out: http://www.weatherstation1.com.
thanks for infoemation , i use work
Hi,
I have arn Arduino y module that I am using to trgger an extractor fan in a shower. I was wondering whether this humidity sensor could be used to simply close the 5v circuit so teh fan runs on until teh humidity is below a set vaue. Is that possible simply?
Hi.recently i conduct sensor circuitry.in source code,i notice that it use \xF8 to display temperature in degree celcius.what is the function of that?
Arduino: 1.8.5 (Windows 10), Board: “Arduino/Genuino Uno”
Sketch uses 4084 bytes (12%) of program storage space. Maximum is 32256 bytes.
Global variables use 239 bytes (11%) of dynamic memory, leaving 1809 bytes for local variables. Maximum is 2048 bytes.
avrdude: ser_open(): can’t open device “\\.\COM1”: The system cannot find the file specified.
Problem uploading to board. See http://www.arduino.cc/en/Guide/Troubleshooting#upload for suggestions.
This report would have more information with
“Show verbose output during compilation”
option enabled in File -> Preferences.
what are the benefits of using dht11
i need dht11 working code for arduino
I found this article to be a whole lot clearer and easier to understand than most, but I do have a few points.
I followed the instructions exactly, wiring was good, code was an exact replica of that given. Everything was correct, but I got -999 error message every time. I was using a three pin sensor, triple checked my wiring against the diagram. I increased the delay time to 3000ms. I was definitely using the correct older version of the library. After throwing out the sensor thinking it faulty, I have since discovered that the diagram above is does not apply to every dht11, that there are some where the pins are in a different order.
My other point is a question about line 3 of the code given: dht DHT;
What does this mean? in every other arduino program I can find that uses additional libraries, the library is called first, then the code goes straight on to initialising the variables and describing the setup. I have not been able to find any other mention of the library name mentioned twice like this. A few people have asked about this, with no answers given. I cant even search for it because I dont know what to search by.
Does this line of code actually do anything?
Awesome website – every content is superb – We have a huge collection of branded Electronics product please have a look here https://webearnorg.blogspot.com/2018/05/electronics-supply-stores-near-me.html
Is it possible to change dht11 to a dht22?
Clear, informative and knowledgeable. Moisture from the air collects on the film and causes changes in the voltage levels between the two plates. This change is then converted into a digital measurement of the air’s relative humidity after taking the air temperature into account.
I have connected dht11 sensor as shown in the image and uploaded the first program but in serial monitor I get output as following
Temperature= 31.00
Humidity=75.00
Temperature= -999.00
Humidity= -999.00
i.e. alternatively I get values and -999
PS:i have followed every step including adding library
Me too. How did you fix this? All I get are
Temperature= -999.00
Humidity= -999.00
Never have gotten a reading.
I gave up on the DHT11 and 22. Buy the SHT31-D. No problem using it on both Arduino Uno and Rasp P13B+ Super easy and very accurate.
Hello
is the temperature in Fahrenheit or Celsius ??
if it is in Fahrenheit how we can convert in Celsius ??
Hi sir,
How to measure humidity value in cold,hot,normal water using Humidity sensor with arduino
You will get 100% humidity if you put the sensor in water and it works. Use an SHT31-D breakout board to detect humidity and temperature. I’m sure you didn’t mean you are going to submerge the sensor. The SHT31-D is more accurate and easier to install and costs about the same as the DHT11 /22 both of which really aren’t accurate at all.
Sir please help!
My lcd is constantly displaying -999C and -999% humiditiy.
i have changed the sensor, checked voltages at each junction,switched pull up resistor, included the exact library available here but couldn’t get the accurate result,
Whereelse can be the issue now?
Ine is set up exactly as you show. I get -999.00 for both humidity and temperature. I have 2 different sensors (both DHT11) and I get the same readings. I even set this up on an RPI 3B+ and the readings were similar. 1.0 temp and humidity. What am I doing wrong?
hello .
i am receiving an error that say’s : error compiling for board arduino/genuino uno
and i can’t find the problem.
what may be the problem?
Buy a SHT31-D. Works great on Arduino UNO and RPI 3B+. Accurate too.
Can it work for computers?
It’s a pity you don’t have a donate button! I’d definitely donate to this superb blog!
I guess for now i’ll settle for bookmarking and adding your RSS feed
to my Google account. I look forward to fresh updates and will talk about this site with
my Facebook group. Talk soon!
I’m curious, how does the accuracy of this setup compare to a home weather station from a manufacturer like the ones shown at wxobservation.com?
Cool tutorial! Keep up the good work.
very helpful post Thank you very much
Can I ask question like which type of that Arduino Board?
Hello,
I’m a bit new to audrino and i started my first project. I found that this tutorial was the most comprehensive out there, which is awesome. One thing that i’m running into a bit of issue on is that im an “400 invalid_request” while attempting to import the DTH library. I was wondering if you could provide a little assistance to get pass this issue. Please see the full error message below.
thank
kenneth
Hi!
I’m wondering if there’s a way to have this working intermittently? I want to moderate the humidity levels in food containers to prevent mould. If this was running off a battery would it last long enough?
You can put the microcontroller in sleep mod and set it to wake up after ex 60 sec. or longer.
Good luck!
Peter
Can you just please let me know how to use both ardiuno and raspberry pi with dht11 sensor. How to interface both
I can not keep my display from blinking the temp and humidity values. It displays the value but blinks back and forth to -999.00. Thanks for the help I’m new
why is it that the dht library that i downloaded was not working? pls im begging you guys help me with this one :(
I get the error
sketch_jan22a.ino:1:17: fatal error: dht.h: No such file or directory
compilation terminated.
when i try to upload. what do i do to fix this?
Dear sir,
it worked just fine for me.
Thank you kindly and please, keep on the great job !!
how to add library DHTLib on Mac??
Hello, I can´t complete the first test without the LCD, it show me an error like this:
code:1:17: error: dht.h: No such file or directory
compilation terminated.
exit status 1
dht.h: No such file or directory
how can i fix this, thank you
When I originally commented I appear to have clicked the -Notify me when new
comments are added- checkbox and now whenever a comment is added
I receive four emails with the exact same comment. There has to be an easy method you are
able to remove me from that service? Thanks!
Your so awesome dude. I owe you a lot! Thanks for the tutorial dude. you’d help many people. keep going! God bless more power. Im from philippines ^_^
Still getting -999.00 on both temperature and humidity with LCD. If I connects ONLY DTH11 to Arduino with serial monitor it works fine, BUT if I connect it to LCD as described above it shows -999.00 In both LCD and serial monitor. It looks like it disables the DTH11 when connected to LCD. It does not work with dely(2000); or any other value.
i don’t know why but the LCD shows me white circles and within them the text is written also the temp and humidity are a constant 0 even with the serial monitor
not circles, squares*
Thanks for sharing!
It’s good idea for projects. I am thinking of building my own weather unit soon. Please can someone help me with a simulation circuit that will show the response graphs of dht11 for temperature and humidity
How would you configure Celsius to Fahrenheit when doing the LCD version? I read others commenting how with out the LCD but not with the code for using the LCD.
My DHT 11 measured -999,0 C, is it a problem with the sensor?
No problem. DTH-11 is a pretty slow sensor.
As many has commented here:
Add a delay of 1500 or 2000 ms and see if that works :)
I’m looking to couple this humidity sensor with a 5V relay to actuate a small on/off valve depending on the humidity level. Essentially, I’d like valve to open when the humidity reading from the sensor goes above, say, 75%, and closes when the reading goes below 60%. Do you have any recommendations?
SIR PLEASE HELP ME I AM IN BSC IT THIRD YEAR AND I HAVE A PROJECT ON SMART AGRICULTURE AND I WANT TO MAKE A PROJECT ARE 5 SENSOR IN THIS PROJECT
HOW TO CONNECT THE SENSOR OF TEMPERTURE HUMIDITY SENSOR TO ARDUNIO AND ASWELL AS ON SCREE AND WITH IOT TO THINGSPESK PLATFORM ONLINE
HELP ME PLEASE
sunil10102488yadav@gmail.com
whatsapp no : 7045837622
how to convert dht11 sensor value to string when sending through mqtt
I need to incorporate the following in the Arduino environment:-
1.LCD fro displaying the readings of the temperature and Humidity,
2.A relay to control the Exhaust and a fan if the humidity is more and the temperature is more respectively,
I am a hobbyist and has certain experience in electronics and wish to adopt programming. So kindly some one can help me to achieve the above goal with codes and probable sketches for the connections.Hope to receive a reply in this respect from your side.
Hi thanks for the project , but i keep getting :
Temperature = 0.00
Humidity = 0.00
tried different pins , analogs as well , but no change
any ideas?
thanks in advance
HELLO, thank you for the big assistance. I’d like to share to u the screenshoot of my serial monitor output… Atleast let me show how it looks like & help me to debug. Big thanks to you
“Temperature = 25.00
Humidity = 52.00
Temperature = -999.00
Humidity = -999.00
Temperature = 25.00
Humidity = 52.00
Temperature = -999.00
Humidity = -999.00
Temperature = 25.00
Humidity = 52.00
Temperature = -999.00
Humidity = -999.00
Temperature = 25.00
Humidity = 52.00
“
You have to adjust the wait time to less to count for the fact that the sensor Only gives an output for a small amount of time so play around with that to get it to work
Hello very interesting
Could you agree if i am rewriting you content in Belajar Dasar Teknisi
Thanks
Hello,
With thanks, I have answer:
Humidity = 0.00
Temperature = 0.00
Humidity = 0.00
Temperature = 0.00
Humidity = 0.00
Temperature = 0.00
Humidity = 0.00
Would You please help?
Hi, I have a problem with this project. I get the value “-999.00” for temperature and humidity.
I know this is an old post but could you be able to use 2 sensors to beable to such on an extract fan if inside rh is higher than outside rh
I need help! The temperature and humidity are not printing on the LCD! Thanks!
Hi,
I am trying to follow your guide but don't have a similar hardware setup with the sensor, do you know if this sensor would work fine?
Thanks
cant install the DHTLib ?
(DHT.temperature * 1.8 + 32)
C:\Users\AppData\Local\Temp\.arduinoIDE-unsaved2024410-10772-w0l7r6.ysbnd\sketch_may10a\sketch_may10a.ino:6:1: error: ‘dht’ does not name a type
dht DHT;
^~~
C:\Users\\AppData\Local\Temp\.arduinoIDE-unsaved2024410-10772-w0l7r6.ysbnd\sketch_may10a\sketch_may10a.ino: In function ‘void loop()’:
C:\Users\andrei\AppData\Local\Temp\.arduinoIDE-unsaved2024410-10772-w0l7r6.ysbnd\sketch_may10a\sketch_may10a.ino:15:13: error: ‘DHT’ was not declared in this scope
int chk = DHT.read11(DHT11_PIN);
^~~
Mehrere Bibliotheken wurden für “LiquidCrystal.h” gefunden
Benutzt: C:\Users\andrei\Documents\Arduino\libraries\LiquidCrystal
Nicht benutzt: C:\Users\andrei\AppData\Local\Arduino15\libraries\LiquidCrystal
exit status 1
Compilation error: ‘dht’ does not name a type